大球吃小球游戏 python
时间: 2023-08-24 14:09:54 浏览: 57
好的,我可以为您提供一些关于用Python开发大球吃小球游戏的指导。
首先,您需要安装Pygame库,这是一个专门用于游戏开发的Python库。您可以在Pygame官方网站上下载并安装它。
接下来,您需要创建游戏窗口。您可以使用Pygame中的display模块来实现。例如:
```python
import pygame
# 初始化Pygame
pygame.init()
# 创建游戏窗口
window = pygame.display.set_mode((800, 600))
# 设置窗口标题
pygame.display.set_caption("大球吃小球游戏")
# 游戏主循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 更新窗口
pygame.display.update()
```
在游戏主循环中,我们使用Pygame的event模块来处理游戏事件,例如退出事件。然后,我们使用Pygame的display模块来更新游戏窗口。
接下来,您需要创建球体对象。您可以使用Pygame的Surface对象来创建球体,然后使用Pygame的draw模块来绘制球体。例如:
```python
import pygame
# 初始化Pygame
pygame.init()
# 创建游戏窗口
window = pygame.display.set_mode((800, 600))
# 创建球体
ball = pygame.Surface((50, 50))
ball.fill((255, 0, 0)) # 红色
ball_rect = ball.get_rect()
ball_rect.center = (400, 300) # 初始位置
# 设置窗口标题
pygame.display.set_caption("大球吃小球游戏")
# 游戏主循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 绘制球体
window.blit(ball, ball_rect)
# 更新窗口
pygame.display.update()
```
在游戏主循环中,我们使用Pygame的blit方法来将球体绘制到窗口上。
最后,您需要实现球体的移动和吃小球的功能。您可以使用Pygame的Rect对象来表示球体的位置和大小,并使用Pygame的colliderect方法来检测球体是否与小球相交。例如:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 创建游戏窗口
window = pygame.display.set_mode((800, 600))
# 创建球体
ball = pygame.Surface((50, 50))
ball.fill((255, 0, 0)) # 红色
ball_rect = ball.get_rect()
ball_rect.center = (400, 300) # 初始位置
# 创建小球
small_balls = []
for i in range(10):
small_ball = pygame.Surface((20, 20))
small_ball.fill((0, 255, 0)) # 绿色
small_ball_rect = small_ball.get_rect()
small_ball_rect.center = (random.randint(0, 800), random.randint(0, 600))
small_balls.append(small_ball_rect)
# 设置窗口标题
pygame.display.set_caption("大球吃小球游戏")
# 游戏主循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 移动球体
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
ball_rect.move_ip(-5, 0)
if keys[pygame.K_RIGHT]:
ball_rect.move_ip(5, 0)
if keys[pygame.K_UP]:
ball_rect.move_ip(0, -5)
if keys[pygame.K_DOWN]:
ball_rect.move_ip(0, 5)
# 检测碰撞
for small_ball_rect in small_balls:
if ball_rect.colliderect(small_ball_rect):
small_balls.remove(small_ball_rect)
ball_rect.inflate_ip(10, 10)
# 绘制球体和小球
window.fill((255, 255, 255)) # 白色背景
window.blit(ball, ball_rect)
for small_ball_rect in small_balls:
window.blit(small_ball, small_ball_rect)
# 更新窗口
pygame.display.update()
```
在游戏主循环中,我们使用Pygame的key模块来检测键盘事件,例如移动球体。然后,我们使用Pygame的Rect对象的move_ip方法来移动球体。我们还使用Pygame的colliderect方法来检测球体是否与小球相交,并使用Pygame的Rect对象的inflate_ip方法来使球体变大。
最后,我们使用Pygame的fill方法来填充背景色,使用Pygame的blit方法来绘制球体和小球,并使用Pygame的update方法来更新窗口。
希望这些指导对您有所帮助,祝您开发愉快!
相关推荐
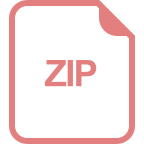
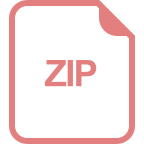
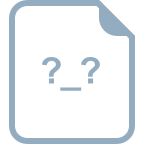











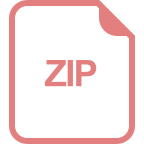