使用c++设计并实现一个教室管理系统,教室需设置不同的类型,系统可以实现对教室信息的添加,修改,删除,查找等功能,教室信息需要以文件方式保存到计算机硬盘中,给出一个教学楼,可以显示该教学楼的所有教室。 基本功能: (1)教室要设置不同的类型,如多媒体教室、智慧教室等; (2)教室信息包括:房间号,教学楼、类型,座位数,卫生责任人,设备责任人等; (3)教室信息要保存在磁盘中; (4)系统界面应该尽量友好,方便使用。
时间: 2023-10-23 19:12:41 浏览: 38
好的,这是一个比较典型的文件管理系统的设计题目,我可以给你提供一个大致的设计思路:
1.定义教室类,包括教室号、教学楼、类型、座位数、卫生责任人、设备责任人等属性。
2.设计教室管理类,实现教室信息的添加、修改、删除、查找等功能。
3.使用文件流将教室信息保存到硬盘中,可以选择使用文本文件或二进制文件。
4.设计主界面,提供友好的操作界面,方便用户进行各种操作。
5.设计教学楼类,包括教学楼的名称、楼层数、包含的教室等信息,实现显示某个教学楼所有教室的功能。
下面是一个可能的代码实现,仅供参考:
```c++
#include <iostream>
#include <fstream>
#include <vector>
using namespace std;
//教室类
class Classroom {
public:
Classroom() {}
Classroom(string roomNum, string building, string type, int seats, string healthManager, string deviceManager) :
roomNum(roomNum), building(building), type(type), seats(seats), healthManager(healthManager), deviceManager(deviceManager) {}
void display() {
cout << "RoomNum: " << roomNum << endl;
cout << "Building: " << building << endl;
cout << "Type: " << type << endl;
cout << "Seats: " << seats << endl;
cout << "Health Manager: " << healthManager << endl;
cout << "Device Manager: " << deviceManager << endl;
}
string roomNum; //教室号
string building; //教学楼
string type; //类型
int seats; //座位数
string healthManager; //卫生责任人
string deviceManager; //设备责任人
};
//教室管理类
class ClassroomManager {
public:
ClassroomManager() {}
void addClassroom() {
Classroom c;
cout << "Enter classroom info: " << endl;
cout << "RoomNum: ";
cin >> c.roomNum;
cout << "Building: ";
cin >> c.building;
cout << "Type: ";
cin >> c.type;
cout << "Seats: ";
cin >> c.seats;
cout << "Health Manager: ";
cin >> c.healthManager;
cout << "Device Manager: ";
cin >> c.deviceManager;
classrooms.push_back(c);
saveToFile();
}
void modifyClassroom() {
string roomNum;
cout << "Enter the room number to modify: ";
cin >> roomNum;
for (int i = 0; i < classrooms.size(); i++) {
if (classrooms[i].roomNum == roomNum) {
cout << "Enter new info: " << endl;
cout << "RoomNum: ";
cin >> classrooms[i].roomNum;
cout << "Building: ";
cin >> classrooms[i].building;
cout << "Type: ";
cin >> classrooms[i].type;
cout << "Seats: ";
cin >> classrooms[i].seats;
cout << "Health Manager: ";
cin >> classrooms[i].healthManager;
cout << "Device Manager: ";
cin >> classrooms[i].deviceManager;
saveToFile();
cout << "Modify success!" << endl;
return;
}
}
cout << "Room number not found!" << endl;
}
void deleteClassroom() {
string roomNum;
cout << "Enter the room number to delete: ";
cin >> roomNum;
for (vector<Classroom>::iterator it = classrooms.begin(); it != classrooms.end(); it++) {
if (it->roomNum == roomNum) {
classrooms.erase(it);
saveToFile();
cout << "Delete success!" << endl;
return;
}
}
cout << "Room number not found!" << endl;
}
void searchClassroom() {
string roomNum;
cout << "Enter the room number to search: ";
cin >> roomNum;
for (int i = 0; i < classrooms.size(); i++) {
if (classrooms[i].roomNum == roomNum) {
classrooms[i].display();
return;
}
}
cout << "Room number not found!" << endl;
}
void displayAll() {
for (int i = 0; i < classrooms.size(); i++) {
classrooms[i].display();
cout << endl;
}
}
//保存教室信息到文件
void saveToFile() {
ofstream outfile("classrooms.txt");
for (int i = 0; i < classrooms.size(); i++) {
outfile << classrooms[i].roomNum << " ";
outfile << classrooms[i].building << " ";
outfile << classrooms[i].type << " ";
outfile << classrooms[i].seats << " ";
outfile << classrooms[i].healthManager << " ";
outfile << classrooms[i].deviceManager << endl;
}
outfile.close();
}
//从文件读取教室信息
void loadFromFile() {
ifstream infile("classrooms.txt");
if (!infile) {
cout << "File not found!" << endl;
return;
}
string roomNum, building, type, healthManager, deviceManager;
int seats;
while (infile >> roomNum >> building >> type >> seats >> healthManager >> deviceManager) {
Classroom c(roomNum, building, type, seats, healthManager, deviceManager);
classrooms.push_back(c);
}
infile.close();
}
private:
vector<Classroom> classrooms;
};
//教学楼类
class Building {
public:
Building(string name, int levels) : name(name), levels(levels) {}
void displayClassrooms(ClassroomManager& cm) {
cout << "Building Name: " << name << endl;
cout << "Levels: " << levels << endl;
cout << "Classrooms: " << endl;
for (int i = 0; i < cm.classrooms.size(); i++) {
if (cm.classrooms[i].building == name) {
cm.classrooms[i].display();
cout << endl;
}
}
}
private:
string name;
int levels;
};
//主函数
int main()
{
ClassroomManager cm;
cm.loadFromFile();
while (true) {
cout << "1. Add Classroom" << endl;
cout << "2. Modify Classroom" << endl;
cout << "3. Delete Classroom" << endl;
cout << "4. Search Classroom" << endl;
cout << "5. Display All Classrooms" << endl;
cout << "6. Display Building Info" << endl;
cout << "0. Exit" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
cm.addClassroom();
break;
case 2:
cm.modifyClassroom();
break;
case 3:
cm.deleteClassroom();
break;
case 4:
cm.searchClassroom();
break;
case 5:
cm.displayAll();
break;
case 6:
cout << "Enter building name: ";
string buildingName;
cin >> buildingName;
Building b(buildingName, 5); //假设每个教学楼都有5层
b.displayClassrooms(cm);
break;
case 0:
return 0;
default:
cout << "Invalid input!" << endl;
break;
}
}
return 0;
}
```
上面的代码只是一个简单的示例,还有很多细节需要考虑,比如输入验证、错误处理、界面设计等,需要根据实际情况进行完善。
相关推荐
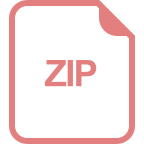
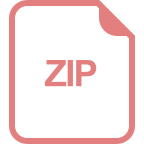
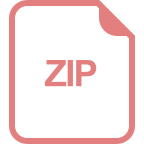

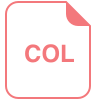

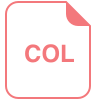
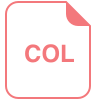
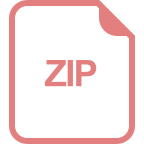
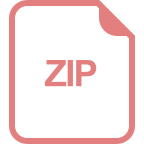
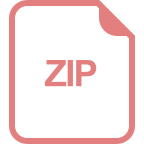
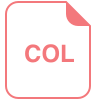
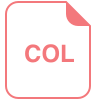
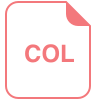
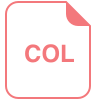
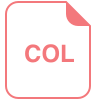
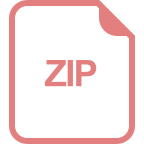
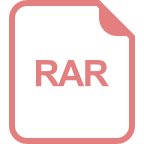
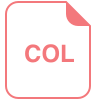