springboot反射注入
时间: 2023-10-14 21:29:54 浏览: 57
在Spring Boot应用程序中使用反射注入可以方便地将依赖项注入到对象中,这可以减少手动创建依赖项实例的工作。以下是一个简单的示例:
1. 创建需要注入依赖项的类
```
public class MyService {
private MyDependency myDependency;
public void setMyDependency(MyDependency myDependency) {
this.myDependency = myDependency;
}
public void doSomething() {
myDependency.doSomethingElse();
}
}
```
2. 创建依赖项类
```
public class MyDependency {
public void doSomethingElse() {
System.out.println("Doing something else...");
}
}
```
3. 在Spring Boot应用程序中使用反射注入
```
@SpringBootApplication
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
// Get instance of MyService class using reflection
Class<?> myServiceClass = Class.forName("com.example.MyService");
Object myServiceObj = myServiceClass.newInstance();
// Get instance of MyDependency class using reflection
Class<?> myDependencyClass = Class.forName("com.example.MyDependency");
Object myDependencyObj = myDependencyClass.newInstance();
// Use reflection to call the setMyDependency method on MyService object
Method setMyDependencyMethod = myServiceClass.getMethod("setMyDependency", myDependencyClass);
setMyDependencyMethod.invoke(myServiceObj, myDependencyObj);
// Use reflection to call the doSomething method on MyService object
Method doSomethingMethod = myServiceClass.getMethod("doSomething");
doSomethingMethod.invoke(myServiceObj);
}
}
```
在这个例子中,我们使用反射获取MyService和MyDependency类的实例,然后使用反射调用setMyDependency和doSomething方法。这样,我们就可以将MyDependency实例注入到MyService实例中,并调用MyService的方法,而不需要手动创建依赖项实例。
相关推荐
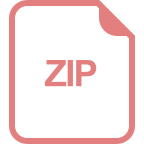
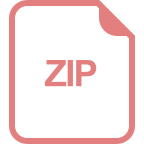














