mybatis 判断 mysql表是否存在,并创建
时间: 2023-08-22 11:09:31 浏览: 253
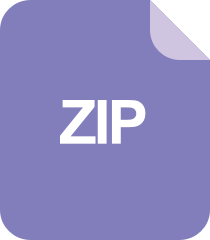
利用MybatisPlus来实现对表的水平分表,全自动,可以首先判断表是否存在,不存在创建表
可以使用 MyBatis-Plus 提供的 `TableInfoHelper` 类来判断 MySQL 表是否存在,并创建表。具体步骤如下:
1. 在 `pom.xml` 中添加 MyBatis-Plus 依赖:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.0</version>
</dependency>
```
2. 在 MyBatis 配置文件中添加配置:
```xml
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource">
<!-- 数据库连接相关配置 -->
</bean>
<bean id="sqlSessionFactory" class="com.baomidou.mybatisplus.extension.spring.MybatisSqlSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<!-- MyBatis 配置文件路径 -->
<property name="configLocation" value="classpath:mybatis-config.xml" />
</bean>
<bean id="sqlSessionTemplate" class="org.mybatis.spring.SqlSessionTemplate">
<constructor-arg index="0" ref="sqlSessionFactory" />
</bean>
```
3. 在代码中调用 `TableInfoHelper` 的 `existTable` 方法来判断表是否存在,并创建表:
```java
@Autowired
private SqlSessionTemplate sqlSessionTemplate;
public void createTableIfNotExist() {
// 获取 Mapper 接口
MybatisMapper mapper = sqlSessionTemplate.getMapper(MybatisMapper.class);
// 判断表是否存在
boolean tableExist = TableInfoHelper.existTable(mapper.getClass());
if (!tableExist) {
// 创建表
TableInfoHelper.createTableIfNotExist(mapper.getClass());
}
}
```
其中,`MybatisMapper` 是你自己定义的 Mapper 接口,需要继承 MyBatis-Plus 提供的 `BaseMapper` 接口。例如:
```java
public interface MybatisMapper extends BaseMapper<MybatisEntity> {
// Mapper 方法
}
```
`MybatisEntity` 是你自己定义的实体类,对应 MySQL 数据库中的表。例如:
```java
@Data
public class MybatisEntity {
private Long id;
private String name;
// 实体类属性
}
```
阅读全文
相关推荐

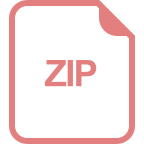
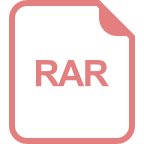
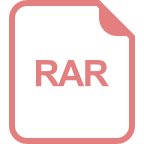
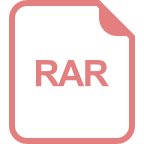
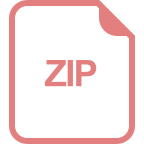
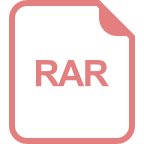
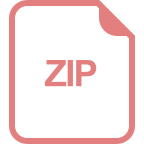
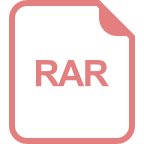
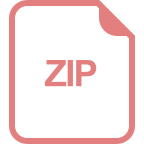
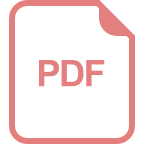
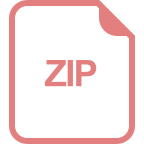
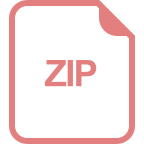
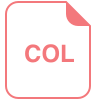
