python实现俄罗斯方块
时间: 2023-07-30 07:12:05 浏览: 41
实现俄罗斯方块可以使用Python中的Pygame库来实现。以下是一个简单的示例代码,可以让你开始学习如何使用Pygame来实现俄罗斯方块。
首先需要安装 Pygame 库,可以使用 pip 命令安装:
```
pip install pygame
```
然后,你可以使用以下代码来实现俄罗斯方块:
```python
import pygame
import random
# 窗口大小
WINDOW_WIDTH = 600
WINDOW_HEIGHT = 720
# 方块大小
BLOCK_SIZE = 30
# 方块颜色
COLORS = [
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255),
(128, 128, 128)
]
# 方块形状
SHAPES = [
[[1, 1, 1, 1]],
[[1, 1, 1], [0, 1, 0]],
[[1, 1, 0], [0, 1, 1]],
[[0, 1, 1], [1, 1, 0]],
[[1, 0, 0], [1, 1, 1]],
[[0, 0, 1], [1, 1, 1]],
[[1, 1], [1, 1]]
]
# 游戏主函数
def main():
# 初始化 Pygame 库
pygame.init()
# 创建游戏窗口
screen = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption('俄罗斯方块')
# 创建字体对象
font = pygame.font.SysFont('SimHei', 24)
# 创建游戏区域
game_area = [[0] * (WINDOW_WIDTH // BLOCK_SIZE) for _ in range(WINDOW_HEIGHT // BLOCK_SIZE)]
# 创建当前方块
current_shape = random.choice(SHAPES)
current_color = random.choice(COLORS)
current_x = (WINDOW_WIDTH // BLOCK_SIZE - len(current_shape[0])) // 2
current_y = 0
# 游戏主循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
return
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
if check_pos(game_area, current_shape, current_color, current_x - 1, current_y):
current_x -= 1
elif event.key == pygame.K_RIGHT:
if check_pos(game_area, current_shape, current_color, current_x + 1, current_y):
current_x += 1
elif event.key == pygame.K_DOWN:
if check_pos(game_area, current_shape, current_color, current_x, current_y + 1):
current_y += 1
elif event.key == pygame.K_UP:
current_shape = rotate_shape(current_shape)
# 绘制游戏区域
for y, row in enumerate(game_area):
for x, color in enumerate(row):
pygame.draw.rect(screen, COLORS[color], (x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 绘制当前方块
draw_shape(screen, current_shape, current_color, current_x, current_y)
# 检查当前方块是否可以下落
if check_pos(game_area, current_shape, current_color, current_x, current_y + 1):
current_y += 1
else:
# 将当前方块放入游戏区域
place_shape(game_area, current_shape, current_color, current_x, current_y)
# 创建新的方块
current_shape = random.choice(SHAPES)
current_color = random.choice(COLORS)
current_x = (WINDOW_WIDTH // BLOCK_SIZE - len(current_shape[0])) // 2
current_y = 0
# 检查是否有行可以消除
lines_cleared = clear_lines(game_area)
# 更新分数
score += lines_cleared * 100
# 绘制分数
score_text = font.render('分数:%d' % score, True, (255, 255, 255))
screen.blit(score_text, (10, 10))
# 刷新屏幕
pygame.display.flip()
# 设置游戏帧率
clock.tick(30)
# 检查方块是否可以放置在游戏区域的指定位置
def check_pos(game_area, shape, color, x, y):
for y_idx, row in enumerate(shape):
for x_idx, cell in enumerate(row):
if cell and (y + y_idx >= len(game_area) or x + x_idx < 0 or x + x_idx >= len(game_area[0]) or game_area[y + y_idx][x + x_idx]):
return False
return True
# 将方块放置在游戏区域的指定位置
def place_shape(game_area, shape, color, x, y):
for y_idx, row in enumerate(shape):
for x_idx, cell in enumerate(row):
if cell:
game_area[y + y_idx][x + x_idx] = COLORS.index(color)
# 绘制方块
def draw_shape(surface, shape, color, x, y):
for y_idx, row in enumerate(shape):
for x_idx, cell in enumerate(row):
if cell:
pygame.draw.rect(surface, color, ((x + x_idx) * BLOCK_SIZE, (y + y_idx) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 旋转方块
def rotate_shape(shape):
return [[shape[y][x] for y in range(len(shape))] for x in range(len(shape[0]) - 1, -1, -1)]
# 清除可以消除的行
def clear_lines(game_area):
lines_cleared = 0
for y, row in enumerate(game_area):
if all(row):
game_area.pop(y)
game_area.insert(0, [0] * (WINDOW_WIDTH // BLOCK_SIZE))
lines_cleared += 1
return lines_cleared
if __name__ == '__main__':
main()
```
这个示例代码可能有点长,但是它提供了一个完整的俄罗斯方块游戏的实现。你可以根据自己的需要对其进行修改和扩展。
相关推荐
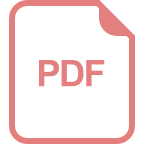
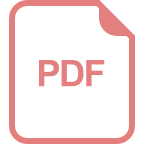












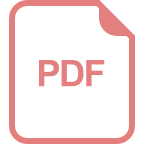