使python实现俄罗斯方块代码
时间: 2023-08-06 19:04:18 浏览: 143
好的,以下是使用Python实现俄罗斯方块的代码:
```python
import pygame
import random
# 游戏窗口大小
WINDOW_WIDTH = 300
WINDOW_HEIGHT = 600
# 游戏区域大小
GAME_WIDTH = 10
GAME_HEIGHT = 20
# 方块大小
BLOCK_SIZE = 30
# 方块移动速度
MOVE_SPEED = 500
# 方块类型及形状
BLOCKS = [
# I型方块
[[[1, 1, 1, 1]]],
# O型方块
[[[1, 1], [1, 1]]],
# T型方块
[[[0, 1, 0], [1, 1, 1]]],
# S型方块
[[[0, 1, 1], [1, 1, 0]]],
# Z型方块
[[[1, 1, 0], [0, 1, 1]]],
# J型方块
[[[1, 0, 0], [1, 1, 1]]],
# L型方块
[[[0, 0, 1], [1, 1, 1]]]
]
# 方块颜色
BLOCK_COLORS = [
(0, 255, 255), # I型方块
(255, 255, 0), # O型方块
(128, 0, 128), # T型方块
(0, 255, 0), # S型方块
(255, 0, 0), # Z型方块
(0, 0, 255), # J型方块
(255, 165, 0) # L型方块
]
# 初始化pygame
pygame.init()
# 创建游戏窗口
screen = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption("俄罗斯方块")
# 加载字体
font = pygame.font.SysFont("SimHei", 30)
# 创建方块类
class Block:
def __init__(self, x, y, block_type):
self.x = x
self.y = y
self.type = block_type
self.color = BLOCK_COLORS[block_type]
self.shape = BLOCKS[block_type][0]
self.rotation = 0
# 旋转方块
def rotate(self):
self.rotation = (self.rotation + 1) % len(self.shape)
self.shape = BLOCKS[self.type][self.rotation]
# 将方块加入游戏区域
def merge(self, game_area):
for i in range(len(self.shape)):
for j in range(len(self.shape[i])):
if self.shape[i][j] == 1:
game_area[self.y + i][self.x + j] = self.type
# 判断方块是否可以移动
def can_move(self, game_area, dx, dy):
for i in range(len(self.shape)):
for j in range(len(self.shape[i])):
if self.shape[i][j] == 1:
x = self.x + j + dx
y = self.y + i + dy
if x < 0 or x >= GAME_WIDTH or y < 0 or y >= GAME_HEIGHT or game_area[y][x] != -1:
return False
return True
# 移动方块
def move(self, game_area, dx, dy):
if self.can_move(game_area, dx, dy):
self.x += dx
self.y += dy
return True
return False
# 绘制方块
def draw(self, screen):
for i in range(len(self.shape)):
for j in range(len(self.shape[i])):
if self.shape[i][j] == 1:
x = self.x + j
y = self.y + i
pygame.draw.rect(screen, self.color, (x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
pygame.draw.rect(screen, (0, 0, 0), (x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE), 1)
# 创建游戏区域
game_area = [[-1] * GAME_WIDTH for i in range(GAME_HEIGHT)]
# 创建当前方块和下一个方块
current_block = Block(3, 0, random.randint(0, len(BLOCKS) - 1))
next_block = Block(3, 0, random.randint(0, len(BLOCKS) - 1))
# 初始化计时器
move_timer = pygame.time.get_ticks()
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
current_block.rotate()
elif event.key == pygame.K_DOWN:
current_block.move(game_area, 0, 1)
elif event.key == pygame.K_LEFT:
current_block.move(game_area, -1, 0)
elif event.key == pygame.K_RIGHT:
current_block.move(game_area, 1, 0)
# 绘制背景
screen.fill((255, 255, 255))
# 绘制游戏区域
for i in range(GAME_HEIGHT):
for j in range(GAME_WIDTH):
if game_area[i][j] != -1:
pygame.draw.rect(screen, BLOCK_COLORS[game_area[i][j]], (j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
pygame.draw.rect(screen, (0, 0, 0), (j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE), 1)
# 绘制当前方块和下一个方块
current_block.draw(screen)
next_block.draw(screen)
# 绘制得分
score_text = font.render("得分:0", True, (0, 0, 0))
screen.blit(score_text, (10, 10))
# 更新屏幕
pygame.display.update()
# 判断是否需要将当前方块加入游戏区域并创建新的方块
if not current_block.move(game_area, 0, 1):
current_block.merge(game_area)
current_block = next_block
next_block = Block(3, 0, random.randint(0, len(BLOCKS) - 1))
# 计时器
if pygame.time.get_ticks() - move_timer > MOVE_SPEED:
current_block.move(game_area, 0, 1)
move_timer = pygame.time.get_ticks()
# 判断是否游戏结束
if not current_block.can_move(game_area, 0, 0):
pygame.quit()
exit()
```
希望这段代码能够帮助到你!
相关推荐
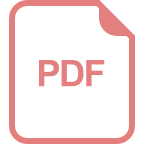














