用python代码实现俄罗斯方块小游戏
时间: 2023-07-27 17:27:55 浏览: 74
好的,以下是一个简单的俄罗斯方块小游戏的Python代码:
```python
import pygame
import random
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRAY = (128, 128, 128)
BLUE = (0, 0, 255)
GREEN = (0, 255, 0)
RED = (255, 0, 0)
YELLOW = (255, 255, 0)
# 定义方块大小
BLOCK_SIZE = 20
# 定义游戏区域大小
SCREEN_WIDTH = 10 * BLOCK_SIZE
SCREEN_HEIGHT = 20 * BLOCK_SIZE
# 定义方块形状
SHAPES = [
[[1, 1, 1],
[0, 1, 0]],
[[1, 1, 0],
[0, 1, 1]],
[[0, 1, 1],
[1, 1, 0]],
[[1, 0, 0],
[1, 1, 1]],
[[0, 0, 1],
[1, 1, 1]],
[[1, 1],
[1, 1]],
[[1, 1, 1, 1]]
]
# 定义方块颜色
COLORS = [
BLUE,
GREEN,
RED,
YELLOW,
WHITE,
GRAY,
BLACK
]
# 初始化pygame
pygame.init()
# 创建游戏窗口
screen = pygame.display.set_mode([SCREEN_WIDTH, SCREEN_HEIGHT])
pygame.display.set_caption("俄罗斯方块")
# 创建时钟对象
clock = pygame.time.Clock()
# 定义方块类
class Block:
def __init__(self, x, y, shape, color):
self.x = x
self.y = y
self.shape = shape
self.color = color
def draw(self):
for row in range(len(self.shape)):
for col in range(len(self.shape[row])):
if self.shape[row][col] == 1:
pygame.draw.rect(screen, self.color, [(self.x + col) * BLOCK_SIZE, (self.y + row) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE])
def move_down(self):
self.y += 1
def move_left(self):
self.x -= 1
def move_right(self):
self.x += 1
def rotate(self):
self.shape = [[self.shape[y][x] for y in range(len(self.shape))] for x in range(len(self.shape[0]) - 1, -1, -1)]
# 定义游戏类
class Game:
def __init__(self):
self.blocks = []
self.current_block = None
self.next_block = Block(0, 0, random.choice(SHAPES), random.choice(COLORS))
self.score = 0
def add_block(self):
self.current_block = self.next_block
self.next_block = Block(0, 0, random.choice(SHAPES), random.choice(COLORS))
self.blocks.append(self.current_block)
def move_down(self):
if self.current_block.y + len(self.current_block.shape) < SCREEN_HEIGHT // BLOCK_SIZE:
self.current_block.move_down()
else:
self.add_block()
self.clear_lines()
def move_left(self):
if self.current_block.x > 0:
self.current_block.move_left()
def move_right(self):
if self.current_block.x + len(self.current_block.shape[0]) < SCREEN_WIDTH // BLOCK_SIZE:
self.current_block.move_right()
def rotate(self):
self.current_block.rotate()
if self.current_block.x + len(self.current_block.shape[0]) > SCREEN_WIDTH // BLOCK_SIZE:
self.current_block.move_left()
if self.current_block.x < 0:
self.current_block.move_right()
def clear_lines(self):
row_counts = [0] * SCREEN_HEIGHT // BLOCK_SIZE
for block in self.blocks:
for row in range(len(block.shape)):
if all(block.shape[row]):
row_counts[block.y + row] += 1
num_lines_cleared = row_counts.count(len(self.blocks[0].shape))
if num_lines_cleared > 0:
self.score += num_lines_cleared * 100
new_blocks = []
for block in self.blocks:
if not any([block.y + row == row_counts.index(len(block.shape)) for row in range(len(block.shape)) if all(block.shape[row])]):
new_blocks.append(block)
else:
del block
self.blocks = new_blocks
def draw(self):
screen.fill(BLACK)
for block in self.blocks:
block.draw()
self.current_block.draw()
pygame.draw.rect(screen, WHITE, [0, 0, SCREEN_WIDTH, SCREEN_HEIGHT], 5)
font = pygame.font.Font(None, 30)
score_text = font.render("Score: " + str(self.score), True, WHITE)
screen.blit(score_text, [10, SCREEN_HEIGHT + 10])
next_text = font.render("Next block:", True, WHITE)
screen.blit(next_text, [SCREEN_WIDTH + 10, 10])
self.next_block.x = SCREEN_WIDTH // BLOCK_SIZE + 1
self.next_block.y = 4
self.next_block.draw()
# 创建游戏对象
game = Game()
# 游戏循环
done = False
while not done:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
game.move_left()
elif event.key == pygame.K_RIGHT:
game.move_right()
elif event.key == pygame.K_DOWN:
game.move_down()
elif event.key == pygame.K_UP:
game.rotate()
# 更新游戏状态
game.move_down()
# 绘制游戏界面
game.draw()
# 刷新显示
pygame.display.flip()
# 控制帧率
clock.tick(10)
# 退出pygame
pygame.quit()
```
这个代码实现了一个简单的俄罗斯方块小游戏,包括方块的移动、旋转和消除等功能。你可以在Pygame上运行它并尝试玩一下。
相关推荐
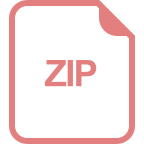
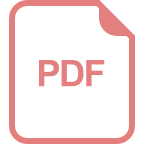
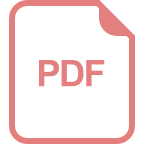
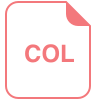
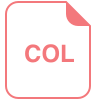
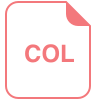
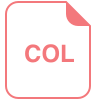
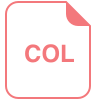









