用python制作俄罗斯方块小游戏
时间: 2023-08-26 19:20:17 浏览: 184
好的,制作俄罗斯方块小游戏可以使用 Python 的 Pygame 模块。以下是一个简单的示例代码:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 游戏区域大小
screen_width = 400
screen_height = 600
# 方块大小
block_size = 20
# 颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
green = (0, 255, 0)
blue = (0, 0, 255)
# 创建游戏窗口
game_display = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption('俄罗斯方块')
# 创建字体对象
font = pygame.font.SysFont(None, 25)
# 定义方块类
class Block:
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
def draw(self):
pygame.draw.rect(game_display, self.color, [self.x, self.y, block_size, block_size])
# 定义方块组类
class BlockGroup:
def __init__(self):
self.blocks = []
def add_block(self, block):
self.blocks.append(block)
def draw(self):
for block in self.blocks:
block.draw()
def move(self, x, y):
for block in self.blocks:
block.x += x
block.y += y
def get_left_edge(self):
left_edge = screen_width
for block in self.blocks:
if block.x < left_edge:
left_edge = block.x
return left_edge
def get_right_edge(self):
right_edge = 0
for block in self.blocks:
if block.x > right_edge:
right_edge = block.x
return right_edge
def get_bottom_edge(self):
bottom_edge = 0
for block in self.blocks:
if block.y > bottom_edge:
bottom_edge = block.y
return bottom_edge
def remove_full_lines(self):
bottom_edge = self.get_bottom_edge()
full_lines = []
for y in range(0, bottom_edge + block_size, block_size):
line_blocks = [block for block in self.blocks if block.y == y]
if len(line_blocks) == screen_width // block_size:
full_lines.append(y)
for block in line_blocks:
self.blocks.remove(block)
if full_lines:
for y in range(max(full_lines) - block_size, -1, -block_size):
line_blocks = [block for block in self.blocks if block.y == y]
for block in line_blocks:
block.y += len(full_lines) * block_size
# 定义游戏主循环
def game_loop():
# 创建方块组对象
block_group = BlockGroup()
# 创建当前方块对象和下一个方块对象
current_block = None
next_block = Block(screen_width // 2 - block_size, 0, random.choice([red, green, blue]))
# 初始化得分和速度
score = 0
speed = 1
# 游戏循环标志
game_exit = False
while not game_exit:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_exit = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
if block_group.get_left_edge() > 0:
block_group.move(-block_size, 0)
elif event.key == pygame.K_RIGHT:
if block_group.get_right_edge() < screen_width - block_size:
block_group.move(block_size, 0)
elif event.key == pygame.K_DOWN:
speed = 5
elif event.key == pygame.K_SPACE:
current_block = None
# 清屏,绘制背景
game_display.fill(white)
# 绘制得分
score_text = font.render('Score: ' + str(score), True, black)
game_display.blit(score_text, [10, 10])
# 绘制下一个方块
next_text = font.render('Next:', True, black)
game_display.blit(next_text, [screen_width - 70, 10])
pygame.draw.rect(game_display, next_block.color, [screen_width - 60, 30, block_size, block_size])
pygame.draw.rect(game_display, next_block.color, [screen_width - 40, 30, block_size, block_size])
pygame.draw.rect(game_display, next_block.color, [screen_width - 20, 30, block_size, block_size])
# 绘制当前方块组
if current_block:
block_group.add_block(current_block)
block_group.draw()
# 如果没有当前方块,则创建新的方块
if not current_block:
current_block = Block(screen_width // 2 - block_size, 0, next_block.color)
next_block = Block(screen_width // 2 - block_size, 0, random.choice([red, green, blue]))
# 如果当前方块到达底部,则将其添加到方块组,清空当前方块
if current_block.y + block_size >= screen_height or \
any([block for block in block_group.blocks if block.x == current_block.x and block.y == current_block.y + block_size]):
block_group.add_block(current_block)
current_block = None
# 移动当前方块
if current_block:
current_block.y += speed
# 移除满行
block_group.remove_full_lines()
if len(block_group.blocks) > 0 and block_group.get_bottom_edge() >= screen_height:
game_display.fill(white)
game_over_text = font.render('Game Over!', True, black)
game_display.blit(game_over_text, [screen_width // 2 - 50, screen_height // 2 - 12])
pygame.display.update()
pygame.time.wait(2000)
game_exit = True
# 更新屏幕
pygame.display.update()
# 控制游戏速度
pygame.time.wait(50)
# 退出 Pygame
pygame.quit()
# 启动游戏循环
game_loop()
```
这个示例代码可能还有一些问题,比如方块组移动时没有判断是否与其他方块重叠,方块的样式比较单一等等,但是可以作为一个简单的起点来制作俄罗斯方块小游戏。
阅读全文
相关推荐
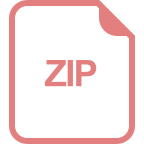
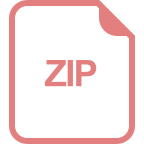

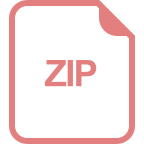



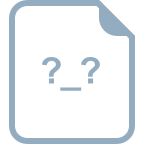
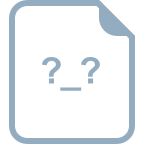
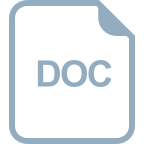





