如何利用python制作俄罗斯方块
时间: 2024-02-10 14:10:43 浏览: 22
要制作俄罗斯方块游戏,可以使用 Python 的 Pygame 库来实现。
首先需要安装 Pygame 库,可以使用 pip 命令在命令行中安装:
```
pip install pygame
```
接下来,可以按照以下步骤来制作俄罗斯方块游戏:
1. 导入 Pygame 库和其他必要的库:
```python
import pygame
import random
```
2. 初始化 Pygame:
```python
pygame.init()
```
3. 设置游戏窗口的大小和标题:
```python
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("Tetris")
```
4. 定义方块的形状和颜色:
```python
shapes = [
[[1, 1, 1], [0, 1, 0]],
[[0, 2, 2], [2, 2, 0]],
[[3, 3, 0], [0, 3, 3]],
[[4, 0, 0], [4, 4, 4]],
[[0, 0, 5], [5, 5, 5]],
[[6, 6], [6, 6]]
]
colors = [
(0, 0, 0),
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255)
]
```
5. 定义方块和游戏板的类:
```python
class Block:
def __init__(self, x, y, shape):
self.x = x
self.y = y
self.shape = shape
self.color = random.randint(1, 6)
self.rotation = 0
class Board:
def __init__(self, width, height):
self.width = width
self.height = height
self.grid = [[0 for y in range(height)] for x in range(width)]
def is_valid_position(self, block):
for x in range(2):
for y in range(3):
if block.shape[block.rotation][y][x] != 0:
if block.x + x < 0 or block.x + x >= self.width or block.y + y >= self.height or self.grid[block.x + x][block.y + y] != 0:
return False
return True
def add_block(self, block):
for x in range(2):
for y in range(3):
if block.shape[block.rotation][y][x] != 0:
self.grid[block.x + x][block.y + y] = block.color
```
6. 定义游戏循环:
```python
board = Board(10, 20)
clock = pygame.time.Clock()
fall_time = 0
current_block = Block(4, 0, shapes[random.randint(0, len(shapes) - 1)])
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 更新方块位置
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
current_block.x -= 1
if not board.is_valid_position(current_block):
current_block.x += 1
elif keys[pygame.K_RIGHT]:
current_block.x += 1
if not board.is_valid_position(current_block):
current_block.x -= 1
elif keys[pygame.K_DOWN]:
current_block.y += 1
if not board.is_valid_position(current_block):
current_block.y -= 1
elif keys[pygame.K_UP]:
current_block.rotation = (current_block.rotation + 1) % len(current_block.shape)
if not board.is_valid_position(current_block):
current_block.rotation = (current_block.rotation - 1) % len(current_block.shape)
# 计算方块下落时间
fall_delay = 1000 - (score * 10)
if fall_time > fall_delay:
current_block.y += 1
if not board.is_valid_position(current_block):
current_block.y -= 1
board.add_block(current_block)
current_block = Block(4, 0, shapes[random.randint(0, len(shapes) - 1)])
fall_time = 0
# 绘制游戏界面
screen.fill((255, 255, 255))
for x in range(board.width):
for y in range(board.height):
pygame.draw.rect(screen, colors[board.grid[x][y]], (x * 30, y * 30, 30, 30), 0)
for x in range(2):
for y in range(3):
if current_block.shape[current_block.rotation][y][x] != 0:
pygame.draw.rect(screen, colors[current_block.color], ((current_block.x + x) * 30, (current_block.y + y) * 30, 30, 30), 0)
pygame.display.update()
# 更新游戏时间
fall_time += clock.tick(60)
```
这样,一个简单的俄罗斯方块游戏就完成了。你可以根据自己的需求进行修改和扩展。
相关推荐
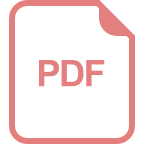
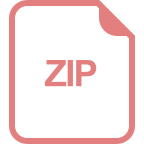
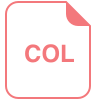
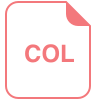
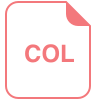
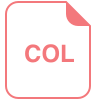
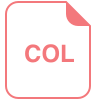









