使用python语言制作俄罗斯方块的示例代码
时间: 2023-07-29 18:07:07 浏览: 48
下面是一个简单的Python代码示例,用于制作俄罗斯方块游戏:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置游戏窗口大小
screen_width = 800
screen_height = 600
game_display = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption('Tetris')
# 定义方块大小和颜色
block_size = 30
color_list = [(0, 0, 0), (255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0), (255, 0, 255), (0, 255, 255), (255, 255, 255)]
# 定义方块形状
tetrominoes = [
[[1, 1, 1], [0, 1, 0]],
[[0, 2, 2], [2, 2, 0]],
[[3, 3], [3, 3]],
[[4, 4, 0], [0, 4, 4]],
[[5, 5, 5, 5]],
[[6, 6, 6], [0, 0, 6]],
[[7, 7, 7], [7, 0, 0]]
]
def draw_block(x, y, color):
pygame.draw.rect(game_display, color, (x, y, block_size, block_size))
def new_block():
global current_block, next_block
current_block = next_block
next_block = random.choice(tetrominoes)
def draw_board(board):
for row in range(20):
for col in range(10):
draw_block(col * block_size, row * block_size, color_list[board[row][col]])
def check_collision(board, block, x, y):
for row in range(len(block)):
for col in range(len(block[0])):
if block[row][col] and board[row+y][col+x]:
return True
return False
def rotate_block(block):
return [[block[y][x] for y in range(len(block))] for x in range(len(block[0])-1, -1, -1)]
# 初始化游戏板
board = [[0 for _ in range(10)] for _ in range(20)]
# 初始化当前方块和下一个方块
current_block = random.choice(tetrominoes)
next_block = random.choice(tetrominoes)
# 初始化方块位置和速度
current_x, current_y = 3, 0
fall_speed = 0.5
fall_time = 0
# 游戏循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT and not check_collision(board, current_block, current_x-1, current_y):
current_x -= 1
elif event.key == pygame.K_RIGHT and not check_collision(board, current_block, current_x+1, current_y):
current_x += 1
elif event.key == pygame.K_DOWN and not check_collision(board, current_block, current_x, current_y+1):
current_y += 1
elif event.key == pygame.K_UP:
current_block = rotate_block(current_block)
if check_collision(board, current_block, current_x, current_y):
current_block = rotate_block(current_block)
elif event.key == pygame.K_SPACE:
while not check_collision(board, current_block, current_x, current_y+1):
current_y += 1
# 更新方块下落位置
fall_time += pygame.time.get_ticks() / 1000
if fall_time >= fall_speed:
fall_time = 0
if not check_collision(board, current_block, current_x, current_y+1):
current_y += 1
else:
# 当前方块落到底部,将其固定在游戏板上
for row in range(len(current_block)):
for col in range(len(current_block[0])):
if current_block[row][col]:
board[row+current_y][col+current_x] = current_block[row][col]
# 消除满行
for row in range(len(board)):
if all(board[row]):
del board[row]
board.insert(0, [0 for _ in range(10)])
# 生成新方块
new_block()
current_x, current_y = 3, 0
# 绘制游戏界面
game_display.fill(color_list[0])
draw_board(board)
for row in range(len(current_block)):
for col in range(len(current_block[0])):
if current_block[row][col]:
draw_block((current_x+col) * block_size, (current_y+row) * block_size, color_list[current_block[row][col]])
pygame.display.update()
```
这个代码示例使用了pygame模块制作了一个简单的俄罗斯方块游戏,并实现了方块的下落、移动、旋转、消除等功能。你可以根据自己的需求进行修改和扩展。
相关推荐
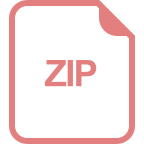
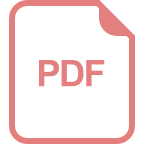
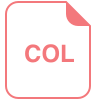
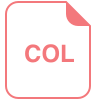
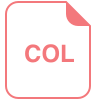
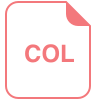







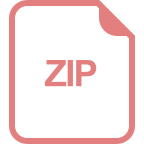
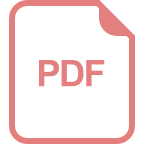
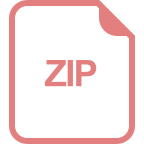
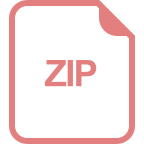