用python制作一个俄罗斯方块
时间: 2023-12-30 07:04:16 浏览: 142
下面是一个简单的 Python 俄罗斯方块游戏示例,供你参考:
```python
import pygame
import random
# 定义一些常量
SCREEN_WIDTH = 640
SCREEN_HEIGHT = 480
BLOCK_SIZE = 20
BOARD_WIDTH = 10
BOARD_HEIGHT = 20
FPS = 60
FONT_SIZE = 24
SHAPE_TYPES = [
((0, 0), (1, 0), (0, 1), (1, 1)), # 方块
((0, 0), (-1, 0), (1, 0), (2, 0)), # 条形
((0, 0), (-1, 0), (0, 1), (1, 1)), # Z 形
((0, 0), (1, 0), (0, 1), (-1, 1)), # 反 Z 形
((0, 0), (0, -1), (0, 1), (1, 0)), # L 形
((0, 0), (0, -1), (0, 1), (-1, 0)), # 反 L 形
((0, 0), (-1, 0), (1, 0), (0, 1)), # T 形
]
# 定义一些颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
CYAN = (0, 255, 255)
MAGENTA = (255, 0, 255)
YELLOW = (255, 255, 0)
# 定义方块类
class Block:
def __init__(self, shape_type, color):
self.shape_type = shape_type
self.color = color
self.x = BOARD_WIDTH // 2
self.y = 0
def rotate(self):
self.shape_type = [(y, -x) for x, y in self.shape_type]
def move(self, dx, dy):
self.x += dx
self.y += dy
def get_blocks(self):
return [(self.x + x, self.y + y) for x, y in self.shape_type]
# 定义游戏主函数
def main():
# 初始化 pygame
pygame.init()
# 设置窗口标题
pygame.display.set_caption("俄罗斯方块")
# 创建游戏窗口
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
# 创建字体对象
font = pygame.font.SysFont(None, FONT_SIZE)
# 创建时钟对象
clock = pygame.time.Clock()
# 创建方块列表
blocks = []
# 创建当前方块
current_block = Block(random.choice(SHAPE_TYPES), random.choice([RED, GREEN, BLUE, CYAN, MAGENTA, YELLOW]))
# 创建游戏结束标志
game_over = False
# 创建得分变量
score = 0
# 游戏循环
while not game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
current_block.move(-1, 0)
if any(x < 0 for x, y in current_block.get_blocks()) or any((x, y) in blocks for x, y in current_block.get_blocks()):
current_block.move(1, 0)
elif event.key == pygame.K_RIGHT:
current_block.move(1, 0)
if any(x >= BOARD_WIDTH for x, y in current_block.get_blocks()) or any((x, y) in blocks for x, y in current_block.get_blocks()):
current_block.move(-1, 0)
elif event.key == pygame.K_UP:
current_block.rotate()
if any(x < 0 or x >= BOARD_WIDTH or y >= BOARD_HEIGHT for x, y in current_block.get_blocks()) or any((x, y) in blocks for x, y in current_block.get_blocks()):
current_block.rotate()
current_block.rotate()
current_block.rotate()
elif event.key == pygame.K_DOWN:
current_block.move(0, 1)
if any(y >= BOARD_HEIGHT for x, y in current_block.get_blocks()) or any((x, y) in blocks for x, y in current_block.get_blocks()):
current_block.move(0, -1)
# 计算下一帧方块位置
next_block = Block(current_block.shape_type, current_block.color)
next_block.move(0, 1)
if any(y >= BOARD_HEIGHT for x, y in next_block.get_blocks()) or any((x, y) in blocks for x, y in next_block.get_blocks()):
for x, y in current_block.get_blocks():
if y < 0:
game_over = True
break
blocks.append((x, y))
current_block = Block(random.choice(SHAPE_TYPES), random.choice([RED, GREEN, BLUE, CYAN, MAGENTA, YELLOW]))
while any((x, y) in blocks for x, y in current_block.get_blocks()):
current_block.move(0, -1)
else:
current_block = next_block
# 绘制背景
screen.fill(BLACK)
# 绘制方块
for x, y in blocks:
pygame.draw.rect(screen, WHITE, (x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 绘制当前方块
for x, y in current_block.get_blocks():
pygame.draw.rect(screen, current_block.color, (x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 绘制得分
score_text = font.render("Score: %d" % score, True, WHITE)
screen.blit(score_text, (10, 10))
# 更新窗口
pygame.display.update()
# 控制帧率
clock.tick(FPS)
# 退出 pygame
pygame.quit()
# 启动游戏
if __name__ == "__main__":
main()
```
这个示例实现了一个简单的俄罗斯方块游戏。玩家可以使用左右箭头键移动方块,上箭头键旋转方块,下箭头键加速方块下落。游戏会根据方块堆叠情况自动清除行并增加得分。游戏结束的条件是方块堆叠到屏幕顶端。注意,这个示例可能还有一些不足之处,需要你自己进行改进。
阅读全文
相关推荐
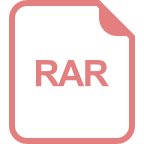
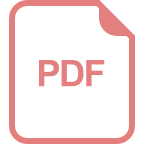







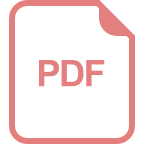
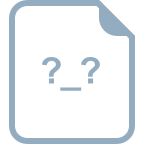
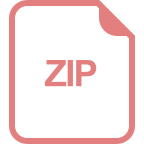