源代码python小俄罗斯方块游戏
时间: 2023-07-25 10:47:40 浏览: 47
以下是一个简单的 Python 小俄罗斯方块游戏的源代码,希望能帮到你:
```python
import pygame
import random
# 初始化 pygame 库
pygame.init()
# 设置游戏窗口大小
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
# 定义方块的大小
block_size = 20
# 定义方块的颜色
colors = [(0, 0, 0), (255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0), (255, 0, 255), (0, 255, 255), (255, 255, 255)]
# 定义方块的形状
shapes = [
[[1, 1, 1],
[0, 1, 0]],
[[0, 2, 2],
[2, 2, 0]],
[[3, 3, 0],
[0, 3, 3]],
[[4, 0, 0],
[4, 4, 4]],
[[0, 0, 5],
[5, 5, 5]],
[[6, 6],
[6, 6]],
[[7, 7, 7, 7]]
]
# 定义方块的初始位置
start_x = screen_width // 2 // block_size * block_size
start_y = 0
# 定义游戏得分
score = 0
# 定义字体
font = pygame.font.SysFont(None, 25)
# 定义方块类
class Block:
def __init__(self, shape, color, x, y):
self.shape = shape
self.color = color
self.x = x
self.y = y
# 向下移动方块
def move_down(self):
self.y += block_size
# 向左移动方块
def move_left(self):
self.x -= block_size
# 向右移动方块
def move_right(self):
self.x += block_size
# 旋转方块
def rotate(self):
self.shape = [[self.shape[j][i] for j in range(len(self.shape))] for i in range(len(self.shape[0]) - 1, -1, -1)]
# 绘制方块
def draw(self):
for i in range(len(self.shape)):
for j in range(len(self.shape[0])):
if self.shape[i][j]:
pygame.draw.rect(screen, self.color, (self.x + j * block_size, self.y + i * block_size, block_size, block_size))
# 判断方块是否碰到边界或其他方块
def is_block_valid(block, board):
for i in range(len(block.shape)):
for j in range(len(block.shape[0])):
if block.shape[i][j]:
x = block.x + j * block_size
y = block.y + i * block_size
if x < 0 or x >= screen_width or y >= screen_height or board[y // block_size][x // block_size]:
return False
return True
# 将方块加入到游戏面板中
def add_block_to_board(block, board):
for i in range(len(block.shape)):
for j in range(len(block.shape[0])):
if block.shape[i][j]:
x = block.x + j * block_size
y = block.y + i * block_size
board[y // block_size][x // block_size] = block.color
# 绘制游戏面板
def draw_board(board):
for i in range(len(board)):
for j in range(len(board[0])):
pygame.draw.rect(screen, colors[board[i][j]], (j * block_size, i * block_size, block_size, block_size), 1)
# 判断是否有消除的行
def get_complete_rows(board):
rows = []
for i in range(len(board)):
if all(board[i]):
rows.append(i)
return rows
# 消除行
def remove_rows(board, rows):
for row in rows:
board.pop(row)
board.insert(0, [0] * (screen_width // block_size))
# 绘制得分
def draw_score(score):
text = font.render("Score: " + str(score), True, (255, 255, 255))
screen.blit(text, (screen_width - text.get_width() - 10, 10))
# 主函数
def main():
# 初始化游戏面板
board = [[0] * (screen_width // block_size) for i in range(screen_height // block_size)]
# 创建一个新的方块
block = Block(random.choice(shapes), random.choice(colors[1:]), start_x, start_y)
# 设置游戏帧率
clock = pygame.time.Clock()
fps = 10
# 游戏循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
block.move_left()
if not is_block_valid(block, board):
block.move_right()
elif event.key == pygame.K_RIGHT:
block.move_right()
if not is_block_valid(block, board):
block.move_left()
elif event.key == pygame.K_DOWN:
block.move_down()
if not is_block_valid(block, board):
block.move_up()
elif event.key == pygame.K_UP:
block.rotate()
if not is_block_valid(block, board):
block.rotate_back()
# 绘制背景
screen.fill((0, 0, 0))
# 绘制游戏面板
draw_board(board)
# 绘制当前方块
block.draw()
# 判断方块是否能够向下移动,如果不能,将其加入到游戏面板中,并创建一个新的方块
if not is_block_valid(block, board):
add_block_to_board(block, board)
rows = get_complete_rows(board)
if rows:
score += len(rows) * 10
remove_rows(board, rows)
block = Block(random.choice(shapes), random.choice(colors[1:]), start_x, start_y)
if not is_block_valid(block, board):
pygame.quit()
quit()
# 绘制得分
draw_score(score)
# 更新游戏画面
pygame.display.update()
# 控制游戏帧率
clock.tick(fps)
if __name__ == "__main__":
main()
```
希望对你有所帮助!
相关推荐
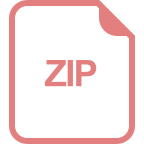














