python俄罗斯方块源代码
时间: 2023-08-29 21:06:58 浏览: 158
这里给您提供一个简单的Python俄罗斯方块源代码,希望对您有帮助:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRAY = (128, 128, 128)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
GREEN = (0, 255, 0)
YELLOW = (255, 255, 0)
# 设置屏幕尺寸
SCREEN_WIDTH = 400
SCREEN_HEIGHT = 500
BLOCK_SIZE = 20
# 创建屏幕对象
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("俄罗斯方块游戏")
# 定义字体
font = pygame.font.SysFont("arial", 20)
# 定义方块
class Block:
def __init__(self, color):
self.color = color
self.shape = random.choice(["I", "J", "L", "O", "S", "T", "Z"])
self.rotation = 0
self.x = SCREEN_WIDTH // 2 - BLOCK_SIZE * 2
self.y = 0
self.blocks = []
self.create_blocks()
# 创建方块
def create_blocks(self):
if self.shape == "I":
self.blocks.append([self.x, self.y])
self.blocks.append([self.x + BLOCK_SIZE, self.y])
self.blocks.append([self.x + BLOCK_SIZE * 2, self.y])
self.blocks.append([self.x + BLOCK_SIZE * 3, self.y])
elif self.shape == "J":
self.blocks.append([self.x, self.y])
self.blocks.append([self.x, self.y + BLOCK_SIZE])
self.blocks.append([self.x + BLOCK_SIZE, self.y + BLOCK_SIZE])
self.blocks.append([self.x + BLOCK_SIZE * 2, self.y + BLOCK_SIZE])
elif self.shape == "L":
self.blocks.append([self.x + BLOCK_SIZE * 2, self.y])
self.blocks.append([self.x, self.y + BLOCK_SIZE])
self.blocks.append([self.x + BLOCK_SIZE, self.y + BLOCK_SIZE])
self.blocks.append([self.x + BLOCK_SIZE * 2, self.y + BLOCK_SIZE])
elif self.shape == "O":
self.blocks.append([self.x, self.y])
self.blocks.append([self.x + BLOCK_SIZE, self.y])
self.blocks.append([self.x, self.y + BLOCK_SIZE])
self.blocks.append([self.x + BLOCK_SIZE, self.y + BLOCK_SIZE])
elif self.shape == "S":
self.blocks.append([self.x + BLOCK_SIZE, self.y])
self.blocks.append([self.x + BLOCK_SIZE * 2, self.y])
self.blocks.append([self.x, self.y + BLOCK_SIZE])
self.blocks.append([self.x + BLOCK_SIZE, self.y + BLOCK_SIZE])
elif self.shape == "T":
self.blocks.append([self.x + BLOCK_SIZE, self.y])
self.blocks.append([self.x, self.y + BLOCK_SIZE])
self.blocks.append([self.x + BLOCK_SIZE, self.y + BLOCK_SIZE])
self.blocks.append([self.x + BLOCK_SIZE * 2, self.y + BLOCK_SIZE])
elif self.shape == "Z":
self.blocks.append([self.x, self.y])
self.blocks.append([self.x + BLOCK_SIZE, self.y])
self.blocks.append([self.x + BLOCK_SIZE, self.y + BLOCK_SIZE])
self.blocks.append([self.x + BLOCK_SIZE * 2, self.y + BLOCK_SIZE])
self.rotate()
# 旋转方块
def rotate(self):
for i in range(len(self.blocks)):
x = self.blocks[i][0]
y = self.blocks[i][1]
if self.shape != "O":
if self.rotation == 0:
self.blocks[i][0] = x + BLOCK_SIZE
self.blocks[i][1] = y - BLOCK_SIZE
elif self.rotation == 1:
self.blocks[i][0] = x + BLOCK_SIZE
self.blocks[i][1] = y + BLOCK_SIZE
elif self.rotation == 2:
self.blocks[i][0] = x - BLOCK_SIZE
self.blocks[i][1] = y + BLOCK_SIZE
elif self.rotation == 3:
self.blocks[i][0] = x - BLOCK_SIZE
self.blocks[i][1] = y - BLOCK_SIZE
# 移动方块
def move(self, dx, dy):
for i in range(len(self.blocks)):
self.blocks[i][0] += dx
self.blocks[i][1] += dy
self.x += dx
self.y += dy
# 绘制方块
def draw(self, surface):
for block in self.blocks:
pygame.draw.rect(surface, self.color, (block[0], block[1], BLOCK_SIZE, BLOCK_SIZE))
# 定义游戏
class Game:
def __init__(self):
self.score = 0
self.game_over = False
self.block = Block(random.choice([RED, BLUE, GREEN, YELLOW]))
self.blocks = []
self.speed = 1
self.clock = pygame.time.Clock()
# 更新游戏
def update(self):
if not self.game_over:
self.block.move(0, BLOCK_SIZE)
if self.check_collision():
self.add_to_blocks()
self.block = Block(random.choice([RED, BLUE, GREEN, YELLOW]))
if self.check_game_over():
self.game_over = True
self.remove_full_rows()
self.speed = min(self.speed + self.score // 10, 10)
self.clock.tick(self.speed)
# 检查碰撞
def check_collision(self):
for block in self.block.blocks:
if block[1] >= SCREEN_HEIGHT - BLOCK_SIZE:
return True
for b in self.blocks:
if block[0] == b[0] and block[1] == b[1]:
return True
return False
# 添加到方块组
def add_to_blocks(self):
for block in self.block.blocks:
self.blocks.append(block)
self.score += 10
# 检查游戏结束
def check_game_over(self):
for block in self.blocks:
if block[1] == 0:
return True
return False
# 移除满行
def remove_full_rows(self):
rows = {}
for block in self.blocks:
if block[1] not in rows:
rows[block[1]] = [block]
else:
rows[block[1]].append(block)
for row in rows:
if len(rows[row]) == SCREEN_WIDTH // BLOCK_SIZE:
for block in rows[row]:
self.blocks.remove(block)
for block in self.blocks:
if block[1] < row:
block[1] += BLOCK_SIZE
self.score += 100
# 绘制游戏
def draw(self, surface):
surface.fill(BLACK)
for block in self.blocks:
pygame.draw.rect(surface, GRAY, (block[0], block[1], BLOCK_SIZE, BLOCK_SIZE))
self.block.draw(surface)
score_text = font.render("Score: " + str(self.score), True, WHITE)
surface.blit(score_text, (10, 10))
if self.game_over:
game_over_text = font.render("Game Over!", True, WHITE)
surface.blit(game_over_text, (SCREEN_WIDTH // 2 - game_over_text.get_width() // 2, SCREEN_HEIGHT // 2 - game_over_text.get_height() // 2))
# 创建游戏对象
game = Game()
# 循环运行游戏
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
game.block.move(-BLOCK_SIZE, 0)
if game.check_collision():
game.block.move(BLOCK_SIZE, 0)
elif event.key == pygame.K_RIGHT:
game.block.move(BLOCK_SIZE, 0)
if game.check_collision():
game.block.move(-BLOCK_SIZE, 0)
elif event.key == pygame.K_UP:
game.block.rotation = (game.block.rotation + 1) % 4
game.block.rotate()
if game.check_collision():
game.block.rotation = (game.block.rotation - 1) % 4
game.block.rotate()
elif event.key == pygame.K_DOWN:
game.speed = 10
game.update()
game.draw(screen)
pygame.display.update()
```
阅读全文
相关推荐
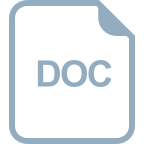
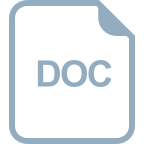
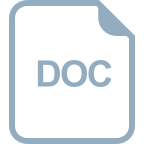
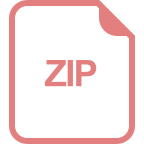
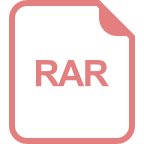
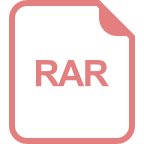




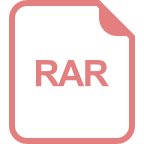
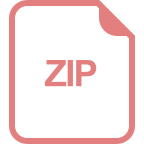
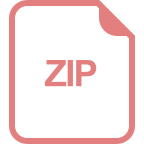


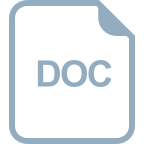
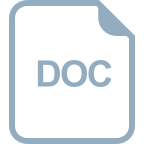