c++ openssl ecc算法大文件加密解密示例代码
时间: 2023-08-26 19:03:50 浏览: 83
下面是一个使用C++和OpenSSL库进行ECC算法大文件加密解密的示例代码:
```c++
#include <openssl/ec.h>
#include <openssl/ecdh.h>
#include <openssl/evp.h>
#include <openssl/pem.h>
#include <openssl/err.h>
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main()
{
// 1. 生成ECC密钥对
EC_KEY *key = EC_KEY_new_by_curve_name(NID_X9_62_prime256v1);
if (key == NULL)
{
cout << "Error: Failed to create ECC key." << endl;
return 1;
}
if (!EC_KEY_generate_key(key))
{
cout << "Error: Failed to generate ECC key." << endl;
EC_KEY_free(key);
return 1;
}
// 2. 保存私钥
string privkey_filename = "ecc_private.pem";
FILE *privkey_file = fopen(privkey_filename.c_str(), "w");
if (privkey_file == NULL)
{
cout << "Error: Failed to create private key file." << endl;
EC_KEY_free(key);
return 1;
}
if (!PEM_write_ECPrivateKey(privkey_file, key, NULL, NULL, 0, NULL, NULL))
{
cout << "Error: Failed to write private key." << endl;
fclose(privkey_file);
EC_KEY_free(key);
return 1;
}
fclose(privkey_file);
// 3. 保存公钥
string pubkey_filename = "ecc_public.pem";
FILE *pubkey_file = fopen(pubkey_filename.c_str(), "w");
if (pubkey_file == NULL)
{
cout << "Error: Failed to create public key file." << endl;
EC_KEY_free(key);
return 1;
}
if (!PEM_write_EC_PUBKEY(pubkey_file, key))
{
cout << "Error: Failed to write public key." << endl;
fclose(pubkey_file);
EC_KEY_free(key);
return 1;
}
fclose(pubkey_file);
// 4. 加密文件
string plaintext_filename = "largefile.txt";
string ciphertext_filename = "largefile.enc";
ifstream plaintext_file(plaintext_filename, ios::in | ios::binary);
if (!plaintext_file.is_open())
{
cout << "Error: Failed to open plaintext file." << endl;
EC_KEY_free(key);
return 1;
}
ofstream ciphertext_file(ciphertext_filename, ios::out | ios::binary);
if (!ciphertext_file.is_open())
{
cout << "Error: Failed to create ciphertext file." << endl;
plaintext_file.close();
EC_KEY_free(key);
return 1;
}
EVP_PKEY *evp_pubkey = EVP_PKEY_new();
if (evp_pubkey == NULL)
{
cout << "Error: Failed to create EVP public key." << endl;
plaintext_file.close();
ciphertext_file.close();
EC_KEY_free(key);
return 1;
}
if (!EVP_PKEY_set1_EC_KEY(evp_pubkey, key))
{
cout << "Error: Failed to set EVP public key." << endl;
plaintext_file.close();
ciphertext_file.close();
EVP_PKEY_free(evp_pubkey);
EC_KEY_free(key);
return 1;
}
EVP_CIPHER_CTX *ctx = EVP_CIPHER_CTX_new();
if (ctx == NULL)
{
cout << "Error: Failed to create cipher context." << endl;
plaintext_file.close();
ciphertext_file.close();
EVP_PKEY_free(evp_pubkey);
EC_KEY_free(key);
return 1;
}
if (!EVP_EncryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, NULL, NULL))
{
cout << "Error: Failed to initialize encryption context." << endl;
plaintext_file.close();
ciphertext_file.close();
EVP_PKEY_free(evp_pubkey);
EC_KEY_free(key);
EVP_CIPHER_CTX_free(ctx);
return 1;
}
unsigned char plaintext_buffer[4096];
unsigned char ciphertext_buffer[4096 + EVP_CIPHER_block_size(EVP_aes_256_cbc())];
int num_bytes_read = 0;
int ciphertext_len = 0;
while ((num_bytes_read = plaintext_file.read((char *)plaintext_buffer, sizeof(plaintext_buffer)).gcount()) > 0)
{
if (!EVP_EncryptInit_ex(ctx, NULL, NULL, NULL, NULL))
{
cout << "Error: Failed to reset encryption context." << endl;
plaintext_file.close();
ciphertext_file.close();
EVP_PKEY_free(evp_pubkey);
EC_KEY_free(key);
EVP_CIPHER_CTX_free(ctx);
return 1;
}
if (!EVP_EncryptInit_ex(ctx, NULL, NULL, EVP_PKEY_get0(evp_pubkey), NULL))
{
cout << "Error: Failed to set public key for encryption context." << endl;
plaintext_file.close();
ciphertext_file.close();
EVP_PKEY_free(evp_pubkey);
EC_KEY_free(key);
EVP_CIPHER_CTX_free(ctx);
return 1;
}
if (!EVP_EncryptUpdate(ctx, ciphertext_buffer, &ciphertext_len, plaintext_buffer, num_bytes_read))
{
cout << "Error: Failed to encrypt plaintext." << endl;
plaintext_file.close();
ciphertext_file.close();
EVP_PKEY_free(evp_pubkey);
EC_KEY_free(key);
EVP_CIPHER_CTX_free(ctx);
return 1;
}
ciphertext_file.write((char *)ciphertext_buffer, ciphertext_len);
}
if (!EVP_EncryptFinal_ex(ctx, ciphertext_buffer, &ciphertext_len))
{
cout << "Error: Failed to finalize encryption." << endl;
plaintext_file.close();
ciphertext_file.close();
EVP_PKEY_free(evp_pubkey);
EC_KEY_free(key);
EVP_CIPHER_CTX_free(ctx);
return 1;
}
ciphertext_file.write((char *)ciphertext_buffer, ciphertext_len);
plaintext_file.close();
ciphertext_file.close();
EVP_PKEY_free(evp_pubkey);
EC_KEY_free(key);
EVP_CIPHER_CTX_free(ctx);
// 5. 解密文件
ifstream ciphertext_file2(ciphertext_filename, ios::in | ios::binary);
if (!ciphertext_file2.is_open())
{
cout << "Error: Failed to open ciphertext file." << endl;
return 1;
}
ofstream plaintext_file2("largefile.dec", ios::out | ios::binary);
if (!plaintext_file2.is_open())
{
cout << "Error: Failed to create plaintext file." << endl;
ciphertext_file2.close();
return 1;
}
EVP_PKEY *evp_privkey = EVP_PKEY_new();
if (evp_privkey == NULL)
{
cout << "Error: Failed to create EVP private key." << endl;
ciphertext_file2.close();
plaintext_file2.close();
return 1;
}
if (!EVP_PKEY_set1_EC_KEY(evp_privkey, key))
{
cout << "Error: Failed to set EVP private key." << endl;
ciphertext_file2.close();
plaintext_file2.close();
EVP_PKEY_free(evp_privkey);
return 1;
}
ctx = EVP_CIPHER_CTX_new();
if (ctx == NULL)
{
cout << "Error: Failed to create cipher context." << endl;
ciphertext_file2.close();
plaintext_file2.close();
EVP_PKEY_free(evp_privkey);
return 1;
}
if (!EVP_DecryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, NULL, NULL))
{
cout << "Error: Failed to initialize decryption context." << endl;
ciphertext_file2.close();
plaintext_file2.close();
EVP_PKEY_free(evp_privkey);
EVP_CIPHER_CTX_free(ctx);
return 1;
}
ciphertext_len = 0;
int plaintext_len = 0;
while ((ciphertext_file2.read((char *)ciphertext_buffer, sizeof(ciphertext_buffer)).gcount()) > 0)
{
if (!EVP_DecryptInit_ex(ctx, NULL, NULL, EVP_PKEY_get0(evp_privkey), NULL))
{
cout << "Error: Failed to set private key for decryption context." << endl;
ciphertext_file2.close();
plaintext_file2.close();
EVP_PKEY_free(evp_privkey);
EVP_CIPHER_CTX_free(ctx);
return 1;
}
if (!EVP_DecryptUpdate(ctx, plaintext_buffer, &plaintext_len, ciphertext_buffer, ciphertext_file2.gcount()))
{
cout << "Error: Failed to decrypt ciphertext." << endl;
ciphertext_file2.close();
plaintext_file2.close();
EVP_PKEY_free(evp_privkey);
EVP_CIPHER_CTX_free(ctx);
return 1;
}
plaintext_file2.write((char *)plaintext_buffer, plaintext_len);
}
if (!EVP_DecryptFinal_ex(ctx, plaintext_buffer, &plaintext_len))
{
cout << "Error: Failed to finalize decryption." << endl;
ciphertext_file2.close();
plaintext_file2.close();
EVP_PKEY_free(evp_privkey);
EVP_CIPHER_CTX_free(ctx);
return 1;
}
plaintext_file2.write((char *)plaintext_buffer, plaintext_len);
ciphertext_file2.close();
plaintext_file2.close();
EVP_PKEY_free(evp_privkey);
EVP_CIPHER_CTX_free(ctx);
cout << "ECC encryption and decryption completed successfully." << endl;
return 0;
}
```
注意:为了简化代码,这里没有进行错误处理和参数检查,实际应用中需要根据需要进行适当的错误处理和参数检查。
相关推荐
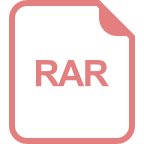
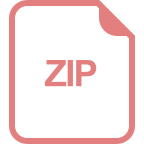











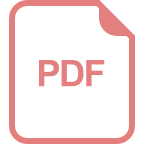
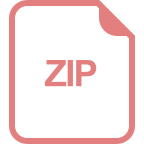
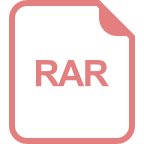