开发一个简单的课表查询系统,实现以下基本功能: 【基本功能】 课表数据文件。每个课表的信息包括:学期、专业班级、时间、科目、教室等基本信息。 能够按给定班级、时间等信息进行查找并显示相关信息。 能够添加、删除或修改课表中的课程信息。 能够按照学期或班级信息对课表进行排序,并存入文件中。 【扩展功能】 可以按照自己对课表查询系统的需求理解进行扩展。比如:(1)权限处理 (2)模糊查询(3)综合查询(4)统计功能等。用C++写出完整代码
时间: 2024-03-24 09:41:37 浏览: 21
很好,这是一个比较具体的问题,我可以为您提供一个基本的实现思路。
首先,我们需要定义一个课程类,用来存储每个课程的信息,包括学期、专业班级、时间、科目、教室等基本信息。类的定义如下:
```cpp
class Course {
public:
string term; // 学期
string className; // 班级名称
string time; // 上课时间
string subject; // 课程名称
string classroom; // 上课教室
bool operator < (const Course& c) const {
// 按照学期和班级名称进行排序
if (term != c.term) return term < c.term;
return className < c.className;
}
};
```
接下来,我们需要一个课表类,用来存储所有的课程信息,并提供相应的操作接口。类的定义如下:
```cpp
class Schedule {
public:
vector<Course> courses; // 所有的课程信息
// 添加课程
void addCourse(const Course& c) {
courses.push_back(c);
}
// 删除课程
void removeCourse(int index) {
courses.erase(courses.begin() + index);
}
// 修改课程
void modifyCourse(int index, const Course& c) {
courses[index] = c;
}
// 按照学期和班级名称排序
void sort() {
std::sort(courses.begin(), courses.end());
}
// 按照班级和时间查询
vector<Course> search(const string& className, const string& time) const {
vector<Course> result;
for (const auto& c : courses) {
if (c.className == className && c.time == time) {
result.push_back(c);
}
}
return result;
}
// 保存到文件
void saveToFile(const string& fileName) const {
ofstream fout(fileName);
for (const auto& c : courses) {
fout << c.term << " " << c.className << " " << c.time << " " << c.subject << " " << c.classroom << endl;
}
fout.close();
}
// 从文件加载
void loadFromFile(const string& fileName) {
courses.clear();
ifstream fin(fileName);
if (!fin) return;
string line;
while (getline(fin, line)) {
stringstream ss(line);
Course c;
ss >> c.term >> c.className >> c.time >> c.subject >> c.classroom;
courses.push_back(c);
}
fin.close();
}
};
```
有了课程类和课表类的定义,我们就可以开始编写主程序了。主程序需要提供一个菜单,让用户选择不同的操作,包括添加、删除、修改、查询、排序、保存和加载等操作。
```cpp
int main() {
Schedule schedule;
while (true) {
cout << "请选择操作:" << endl;
cout << "1. 添加课程" << endl;
cout << "2. 删除课程" << endl;
cout << "3. 修改课程" << endl;
cout << "4. 查询课程" << endl;
cout << "5. 排序课程" << endl;
cout << "6. 保存课表" << endl;
cout << "7. 加载课表" << endl;
cout << "0. 退出程序" << endl;
int choice;
cin >> choice;
if (choice == 0) {
break;
} else if (choice == 1) {
Course c;
cout << "请输入学期、班级、时间、科目、教室:" << endl;
cin >> c.term >> c.className >> c.time >> c.subject >> c.classroom;
schedule.addCourse(c);
} else if (choice == 2) {
cout << "请输入要删除的课程的编号:" << endl;
int index;
cin >> index;
schedule.removeCourse(index);
} else if (choice == 3) {
cout << "请输入要修改的课程的编号:" << endl;
int index;
cin >> index;
Course c;
cout << "请输入学期、班级、时间、科目、教室:" << endl;
cin >> c.term >> c.className >> c.time >> c.subject >> c.classroom;
schedule.modifyCourse(index, c);
} else if (choice == 4) {
cout << "请输入班级和时间:" << endl;
string className, time;
cin >> className >> time;
vector<Course> result = schedule.search(className, time);
for (const auto& c : result) {
cout << c.term << " " << c.className << " " << c.time << " " << c.subject << " " << c.classroom << endl;
}
} else if (choice == 5) {
schedule.sort();
} else if (choice == 6) {
cout << "请输入文件名:" << endl;
string fileName;
cin >> fileName;
schedule.saveToFile(fileName);
} else if (choice == 7) {
cout << "请输入文件名:" << endl;
string fileName;
cin >> fileName;
schedule.loadFromFile(fileName);
} else {
cout << "无效的操作,请重新输入!" << endl;
}
}
return 0;
}
```
以上就是一个简单的课表查询系统的实现。如果需要扩展功能,可以根据具体需求进行修改和添加。
相关推荐
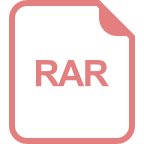
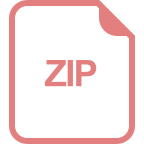












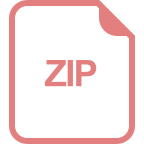