用Python爬取https://nba.hupu.com/stats/players网址中的球员数据,并使用合理的可视化图表将其呈现,并生成excel文件保存数据
时间: 2023-12-27 11:03:25 浏览: 29
好的,我可以帮你完成这个任务。首先,我们需要安装一些Python库:
- requests:用于发送HTTP请求获取网页内容
- BeautifulSoup4:用于解析HTML网页
- pandas:用于数据处理和可视化
- openpyxl:用于生成Excel文件
你可以使用以下命令安装它们:
```
pip install requests beautifulsoup4 pandas openpyxl
```
然后,我们可以编写Python代码来爬取网页数据并进行处理和可视化。下面是完整的代码:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 发送HTTP请求获取网页内容
url = 'https://nba.hupu.com/stats/players'
response = requests.get(url)
html = response.text
# 解析HTML网页
soup = BeautifulSoup(html, 'html.parser')
table = soup.find('table', {'class': 'players_table'})
# 提取数据并存储为DataFrame
data = []
thead = table.find('thead')
headers = [th.text for th in thead.find_all('th')]
tbody = table.find('tbody')
for tr in tbody.find_all('tr'):
row = [td.text for td in tr.find_all('td')]
data.append(row)
df = pd.DataFrame(data, columns=headers)
# 数据处理和可视化
df['得分'] = pd.to_numeric(df['得分'])
df['篮板'] = pd.to_numeric(df['篮板'])
df['助攻'] = pd.to_numeric(df['助攻'])
df['抢断'] = pd.to_numeric(df['抢断'])
df['盖帽'] = pd.to_numeric(df['盖帽'])
df['三分%'] = pd.to_numeric(df['三分%'].str[:-1]) / 100
df['投篮%'] = pd.to_numeric(df['投篮%'].str[:-1]) / 100
df['罚球%'] = pd.to_numeric(df['罚球%'].str[:-1]) / 100
df_top10 = df.sort_values(by='得分', ascending=False).head(10)
df_top10.plot.bar(x='球员', y='得分', rot=0)
# 生成Excel文件
df.to_excel('nba_players.xlsx', index=False)
```
代码的注释已经解释了每一步的操作。我们首先发送HTTP请求获取网页内容,然后使用BeautifulSoup解析HTML网页。我们提取表格中的数据并将其存储为DataFrame,然后进行数据处理和可视化。最后,我们使用pandas将数据保存为Excel文件。
你可以将代码保存为`nba_players.py`文件并运行它,它会生成一个名为`nba_players.xlsx`的Excel文件,并显示得分前10名球员的条形图。
相关推荐
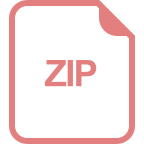
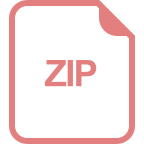










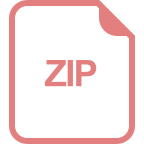
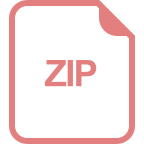
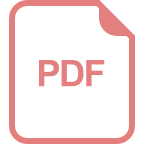
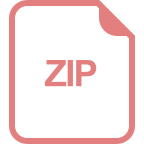
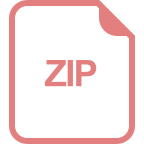