用Matlab写一个做图像平滑的程序,不使用库函数
时间: 2023-12-11 07:02:34 浏览: 27
好的,我可以提供一个基于卷积的图像平滑程序的思路和 Matlab 代码实现。同样地,我们需要使用高斯核或者均值核对图像进行平滑处理。这里我给你提供一个 3x3 的均值核,即每个元素都是 1/9。
```matlab
kernel = [1/9, 1/9, 1/9;
1/9, 1/9, 1/9;
1/9, 1/9, 1/9];
```
接下来,我们需要读取一张图片并将其转为灰度图像。这里使用 Matlab 自带的 `imread` 和 `rgb2gray` 函数来读取和处理图像。
```matlab
img = imread('test.jpg');
gray_img = rgb2gray(img);
[height, width] = size(gray_img);
```
然后,我们对每个像素点进行卷积操作。这里需要注意的是,对于边缘像素点,我们需要进行特殊处理。
```matlab
result = zeros(height, width);
for x = 2 : height - 1
for y = 2 : width - 1
sum = 0;
for i = 1 : 3
for j = 1 : 3
sum = sum + double(gray_img(x + i - 2, y + j - 2)) * kernel(i, j);
end
end
result(x, y) = sum;
end
end
% 处理边缘像素点
for x = 1 : height
result(x, 1) = gray_img(x, 1);
result(x, width) = gray_img(x, width);
end
for y = 1 : width
result(1, y) = gray_img(1, y);
result(height, y) = gray_img(height, y);
end
```
最后,我们将处理后的图像保存。
```matlab
result = uint8(result);
imwrite(result, 'result.jpg');
```
完整代码如下:
```matlab
% 定义卷积核
kernel = [1/9, 1/9, 1/9;
1/9, 1/9, 1/9;
1/9, 1/9, 1/9];
% 读取图像并转为灰度图像
img = imread('test.jpg');
gray_img = rgb2gray(img);
[height, width] = size(gray_img);
% 进行卷积操作
result = zeros(height, width);
for x = 2 : height - 1
for y = 2 : width - 1
sum = 0;
for i = 1 : 3
for j = 1 : 3
sum = sum + double(gray_img(x + i - 2, y + j - 2)) * kernel(i, j);
end
end
result(x, y) = sum;
end
end
% 处理边缘像素点
for x = 1 : height
result(x, 1) = gray_img(x, 1);
result(x, width) = gray_img(x, width);
end
for y = 1 : width
result(1, y) = gray_img(1, y);
result(height, y) = gray_img(height, y);
end
% 保存处理后的图像
result = uint8(result);
imwrite(result, 'result.jpg');
```
相关推荐
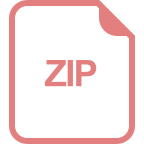
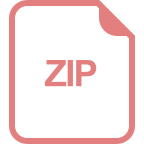












