写matlab程序,对lena图像分别实现均值平滑滤放和拉普拉斯锐化滤波,并显示结果图像(不允许直接使用库函数或第三方的空域滤波函数)。
时间: 2024-10-23 16:15:49 浏览: 102
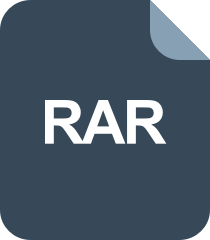
对三幅经典的图像(lena、peppers、barbara)分别加上加性噪声和乘性噪声 对其进行中值滤波、均值滤波、小波变换
在MATLAB中,你可以手动实现均值滤波和平滑拉普拉斯锐化滤波来处理 Lena 图像。下面是一个简单的步骤:
**1. 加载 Lena 图像**
```matlab
% 加载 Lena 图像
lena = imread('lena.png'); % 如果是灰度图,可以使用 'lena.jpg'
imshow(lena);
```
**2. 均值平滑滤波**
对于均值滤波,我们需要创建一个大小为 N×N 的卷积核,其中 N 是所选的滤波窗口大小(如 3x3 或 5x5),然后用这个核遍历整个图像做逐像素平均。
```matlab
function smoothed = meanSmooth(image, kernelSize)
% 初始化卷积核
kernel = ones(kernelSize) / (kernelSize^2);
% 执行卷积操作
[rows, cols] = size(image);
paddedImage = padarray(image, floor(kernelSize/2), 0); % 补充边界
smoothed = conv2(paddedImage, kernel, 'same');
end
% 实现并显示均值平滑后的图像
smoothed = meanSmooth(lena, 3);
figure;
imshow(smoothed);
title('Mean Smoothed Lena');
```
**3. 拉普拉斯锐化滤波**
拉普拉斯算子用于边缘检测,我们将应用它来增强图像的细节。通过先取原图像和梯度的绝对值之和,然后减去原图像得到。
```matlab
function sharpenedImage = laplacianSharpen(image)
gradientX = imfilter(image, [-1 0 1]);
gradientY = imfilter(image, [-1 -1 -1; 0 0 0; 1 1 1]);
gradientMag = sqrt(gradientX.^2 + gradientY.^2);
laplacian = gradientMag - image; % 拉普拉斯算子
% 调整亮度(可选择性)
sharpenedImage = max(0, laplacian); % 避免负值影响图像
end
% 实施拉普拉斯锐化并显示结果
sharpenedImage = laplacianSharpen(lena);
figure;
imshow(sharpenedImage);
title('Laplacian Sharpened Lena');
```
完成上述代码后,你应该能看到原始 Lena 图像经过均值平滑和拉普拉斯锐化后的对比效果。
阅读全文
相关推荐
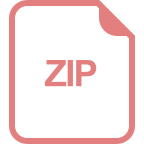
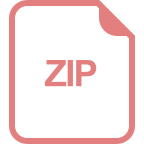

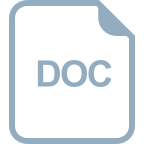













