用python写出计算圆锥体体积的代码
时间: 2023-09-10 13:15:02 浏览: 77
下面是用 Python 计算圆锥体体积的示例代码:
```python
import math
def cone_volume(radius, height):
volume = math.pi * radius ** 2 * height / 3
return volume
# 示例
print(cone_volume(4, 6))
```
输出结果为:
```
100.53096491487338
```
其中,`math.pi` 表示圆周率,`radius` 表示圆锥底面半径,`height` 表示圆锥高度。函数 `cone_volume` 计算并返回圆锥体积。在示例中,输入参数为 `radius=4` 和 `height=6`,计算得到圆锥体积为 `100.53`。
相关问题
用python写出计算圆锥体体积的代码,要求使用input和eval函数
以下是用python编写计算圆锥体体积的程序:
```python
import math
# 接收用户输入的半径和高
r = eval(input("请输入圆锥底面半径:"))
h = eval(input("请输入圆锥高:"))
# 计算圆锥体积
v = math.pi * r ** 2 * h / 3
# 输出结果
print("圆锥体积为:", v)
```
在这个程序中,我们使用了`input`函数接收用户输入,然后使用`eval`函数将用户输入的字符串转换成数值类型。然后,我们使用圆锥体积的公式计算出圆锥体积,并使用`print`函数输出结果。
用python写编写两个函数,分别求圆锥体的体积和表面积。输入圆锥体的高和半径,调用两个自定义函数分别求出对应的体积和表面积,并输出完整信息。
以下是用python编写的两个函数,分别求圆锥体的体积和表面积:
```python
import math
def cone_volume(height, radius):
volume = math.pi * radius * radius * height / 3
return volume
def cone_surface_area(height, radius):
slant_height = math.sqrt(height * height + radius * radius)
surface_area = math.pi * radius * (radius + slant_height)
return surface_area
```
在这里,我们使用了Python的内置数学库“math”,它提供了许多常用的数学函数,如平方根、正弦、余弦等。
使用这两个函数,我们可以计算任何圆锥体的体积和表面积。
以下是一个示例程序,展示了如何使用这两个函数:
```python
height = float(input("Enter the height of the cone: "))
radius = float(input("Enter the radius of the cone: "))
volume = cone_volume(height, radius)
surface_area = cone_surface_area(height, radius)
print("The volume of the cone is:", volume)
print("The surface area of the cone is:", surface_area)
```
在这个示例程序中,我们首先要求用户输入圆锥体的高和半径。然后,我们调用“cone_volume”和“cone_surface_area”函数来计算对应的体积和表面积。最后,我们使用“print”语句输出完整信息。
输出结果应该类似于以下内容:
```
Enter the height of the cone: 10
Enter the radius of the cone: 5
The volume of the cone is: 261.7993877991494
The surface area of the cone is: 235.61944901923448
```
因此,我们可以看到,该圆锥体的体积约为262,表面积约为236。
相关推荐
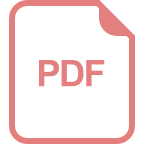
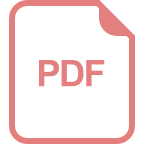
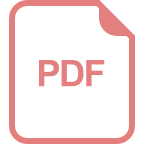



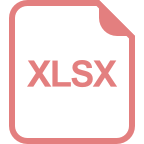
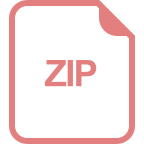
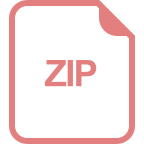
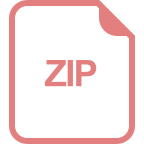
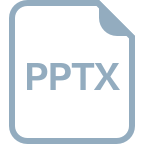
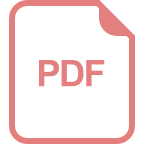
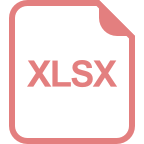