flownet中correlation代码
时间: 2024-01-03 19:02:50 浏览: 72
以下是使用PyTorch实现的FlowNet中的correlation代码:
```python
import torch
import torch.nn.functional as F
class CorrelationLayer(torch.nn.Module):
def __init__(self, max_disp=40):
super(CorrelationLayer, self).__init__()
self.max_disp = max_disp
def forward(self, x1, x2):
b, c, h, w = x1.shape
# pad input tensors
padding = self.max_disp
x1 = F.pad(x1, (padding, padding, padding, padding))
x2 = F.pad(x2, (padding, padding, padding, padding))
# create empty output tensor
out = torch.zeros((b, (2 * self.max_disp + 1)**2, h, w)).to(x1.device)
# loop over all displacements
for i in range(-self.max_disp, self.max_disp+1):
for j in range(-self.max_disp, self.max_disp+1):
# shift x2 by displacement
x2_shifted = x2[:, :, padding+i:h+padding+i, padding+j:w+padding+j]
# compute correlation between x1 and shifted x2
corr = torch.sum(x1 * x2_shifted, dim=1)
# set correlation values outside of valid range to 0
corr[:, :, :i+padding, :] = 0
corr[:, :, :, :j+padding] = 0
corr[:, :, -i-padding:, :] = 0
corr[:, :, :, -j-padding:] = 0
# add correlation values to output tensor
out[:, (i+self.max_disp)*(2*self.max_disp+1) + (j+self.max_disp), :, :] = corr
return out
```
该代码定义了一个名为CorrelationLayer的PyTorch模块,它接受两个输入张量x1和x2,并计算它们之间的相关性。为了处理边缘情况,输入张量被填充了一些额外的像素。输出张量的形状为(b, (2 * max_disp + 1)^2, h, w),其中b是批量大小,h和w是输入张量的高度和宽度,max_disp是允许的最大位移。输出张量的每个通道对应于x1和x2之间的一个位移。在计算相关性时,超出有效范围的值被设置为0。
阅读全文
相关推荐
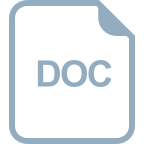
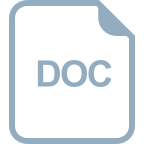
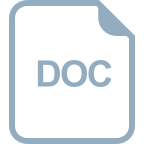


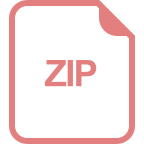
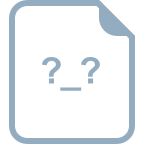
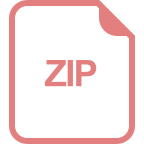
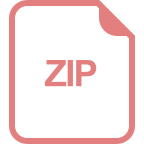
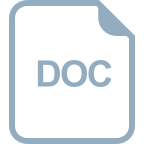
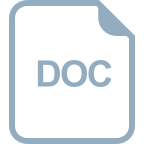
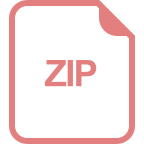
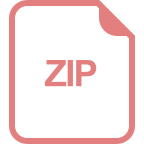
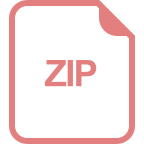
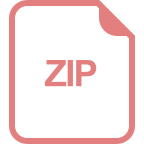
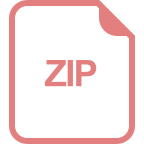
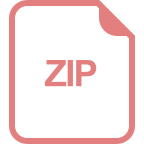
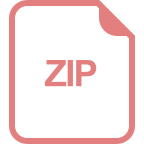
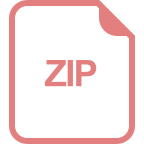