qt在ui端用chartview,接受单片机发来的温湿度数据并绘制折线图
时间: 2024-03-18 16:39:35 浏览: 15
好的,我可以为您解答这个问题。首先,您需要在Qt的UI界面中添加一个ChartView控件,这个控件可以用来绘制折线图。
接下来,您需要通过串口通信方式从单片机中接收到温湿度数据。您可以使用Qt的QSerialPort类来实现串口通信。
一旦您收到了温湿度数据,您可以将其存储在一个数据结构中,例如一个QVector对象。然后,您可以使用QChart类来创建一个折线图,并将数据传递给它以进行绘制。
以下是一个简单的示例代码,它演示了如何使用Qt的ChartView控件来绘制从单片机接收到的温湿度数据的折线图:
```cpp
#include <QtCharts>
#include <QtSerialPort>
#include <QVector>
// Define a data structure to store temperature and humidity readings
struct Reading
{
qreal temperature;
qreal humidity;
};
class MyWidget : public QWidget
{
public:
MyWidget() : QWidget()
{
// Create a chart and add it to a chart view
QChart *chart = new QChart();
QChartView *chartView = new QChartView(chart, this);
setCentralWidget(chartView);
// Create a series to hold the temperature data
QLineSeries *temperatureSeries = new QLineSeries();
temperatureSeries->setName("Temperature");
chart->addSeries(temperatureSeries);
// Create a series to hold the humidity data
QLineSeries *humiditySeries = new QLineSeries();
humiditySeries->setName("Humidity");
chart->addSeries(humiditySeries);
// Create the X and Y axes for the chart
QDateTimeAxis *timeAxis = new QDateTimeAxis();
timeAxis->setTickCount(10);
timeAxis->setFormat("hh:mm:ss");
chart->addAxis(timeAxis, Qt::AlignBottom);
temperatureSeries->attachAxis(timeAxis);
humiditySeries->attachAxis(timeAxis);
QValueAxis *valueAxis = new QValueAxis();
valueAxis->setLabelFormat("%.1f");
chart->addAxis(valueAxis, Qt::AlignLeft);
temperatureSeries->attachAxis(valueAxis);
humiditySeries->attachAxis(valueAxis);
// Set up the serial port to receive data from the microcontroller
QSerialPort *serialPort = new QSerialPort(this);
serialPort->setPortName("COM5"); // Replace with the actual port name
serialPort->setBaudRate(9600);
serialPort->setDataBits(QSerialPort::Data8);
serialPort->setParity(QSerialPort::NoParity);
serialPort->setStopBits(QSerialPort::OneStop);
serialPort->setFlowControl(QSerialPort::NoFlowControl);
serialPort->open(QIODevice::ReadOnly);
connect(serialPort, &QSerialPort::readyRead, this, &MyWidget::readData);
}
private:
QVector<Reading> m_readings;
void readData()
{
QByteArray data = serialPort->readAll();
QString str(data);
// Parse the temperature and humidity values from the received data
qreal temperature = 0.0;
qreal humidity = 0.0;
// Code to parse the temperature and humidity values from the received data goes here
// Add the new reading to the vector
m_readings.append({ temperature, humidity });
// Update the chart with the new data
QLineSeries *temperatureSeries = chart->series()[0];
QLineSeries *humiditySeries = chart->series()[1];
for (int i = 0; i < m_readings.size(); ++i)
{
Reading reading = m_readings[i];
temperatureSeries->append(QDateTime::currentDateTime().toMSecsSinceEpoch(), reading.temperature);
humiditySeries->append(QDateTime::currentDateTime().toMSecsSinceEpoch(), reading.humidity);
}
}
QSerialPort *serialPort;
QChart *chart;
};
```
这个示例代码只是一个简单的演示,其中有一些细节需要自己实现。例如,您需要编写代码来从串口接收数据并将其解析为温湿度值。您还需要根据自己的需求调整图表的样式和布局。
相关推荐
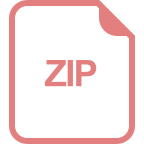














