帮我写一个Python程序,关于统计英文中单词出现的次数并按降序输出。
时间: 2023-06-11 19:08:15 浏览: 83
当然可以,以下是代码实现:
```python
import string
# 打开文件
with open("file.txt", "r") as file:
data = file.read().lower() # 转换为小写字母
# 去掉标点符号
data = data.translate(str.maketrans("", "", string.punctuation))
# 将字符串转换为单词列表
words = data.split()
# 统计单词出现次数
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 按降序输出单词及出现次数
for word, count in sorted(word_count.items(), key=lambda x: x[1], reverse=True):
print(word, count)
```
其中,我们首先打开文件,然后将文件中的所有字母转换为小写字母,去掉标点符号,将字符串转换为单词列表。接着,我们使用字典来统计每个单词出现的次数。最后,我们按降序输出单词及其出现次数,使用 `sorted` 函数和 `lambda` 表达式来实现。
相关问题
要求:用字典编写一个程序,用户输入英文句子,统计每个单词出现的次数,按单词字母排降序输出。
好的,以下是程序的代码:
```python
sentence = input("请输入英文句子:")
# 将句子按空格分割成单词列表
words = sentence.split()
# 统计每个单词出现的次数
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 按单词字母排序并降序输出
sorted_words = sorted(word_count.items(), key=lambda x: x[0], reverse=True)
for word, count in sorted_words:
print(word, count)
```
运行程序后,用户输入英文句子,程序会将句子按空格分割成单词列表,然后统计每个单词出现的次数,最后按单词字母排序并降序输出。
假设当前文件夹下有一个英文的文本文件hamlet.txt,编写程序,统计该英文中单词的出现次数,并将出现次数最多的前20个单词及出现次数按降序显示在屏幕的同时也存到python3.csv文件中
好的,我可以帮你解决这个问题。以下是Python代码:
```python
import re
from collections import Counter
import csv
# 读取文本文件
with open('hamlet.txt', 'r') as f:
text = f.read()
# 去除标点符号和换行符
text = re.sub(r'[^\w\s]', '', text)
text = re.sub(r'\n', ' ', text)
# 统计单词出现次数
words = text.split()
word_counts = Counter(words)
# 获取出现次数最多的前20个单词
top_words = word_counts.most_common(20)
# 输出结果
print('出现次数最多的前20个单词:')
for word, count in top_words:
print(f'{word}: {count}')
# 将结果存储到CSV文件中
with open('python3.csv', 'w', newline='') as f:
writer = csv.writer(f)
writer.writerow(['单词', '出现次数'])
for word, count in top_words:
writer.writerow([word, count])
```
这个程序会读取当前文件夹下的hamlet.txt文件,统计其中单词的出现次数,并将出现次数最多的前20个单词及其出现次数按降序显示在屏幕上,并存储到python3.csv文件中。
相关推荐
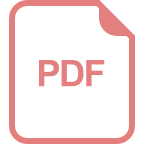
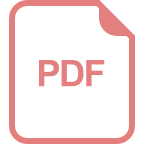













