定义一个表示三维空间坐标点的类,并对下列运算符重载,主函数定义类对象并调用重载的运算符。 (1) 输出流运算符<<:按(x,y,z)格式输出该点坐标(坐标为整型)。 (2) 关系运算符>:如果A点到原点(0,0,0)的距离大于B点到原点的距离,则A>B为真,否则为假。
时间: 2024-03-27 16:35:07 浏览: 10
好的,我理解了你的要求。以下是实现该类以及对应的运算符重载的示例代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
class Point3D {
public:
Point3D(int x, int y, int z) : m_x(x), m_y(y), m_z(z) {}
friend ostream& operator<<(ostream& os, const Point3D& p) {
os << "(" << p.m_x << "," << p.m_y << "," << p.m_z << ")";
return os;
}
bool operator>(const Point3D& other) const {
int distance1 = sqrt(m_x * m_x + m_y * m_y + m_z * m_z);
int distance2 = sqrt(other.m_x * other.m_x + other.m_y * other.m_y + other.m_z * other.m_z);
return distance1 > distance2;
}
private:
int m_x;
int m_y;
int m_z;
};
int main() {
Point3D p1(1, 2, 3);
Point3D p2(4, 5, 6);
cout << "p1: " << p1 << endl;
cout << "p2: " << p2 << endl;
if (p1 > p2) {
cout << "p1 is farther from the origin than p2" << endl;
}
else {
cout << "p2 is farther from the origin than p1" << endl;
}
return 0;
}
```
主函数中定义了两个 Point3D 类对象 p1 和 p2,并对它们进行了输出以及 > 运算符的比较。请注意,> 运算符被定义为成员函数,因此它需要一个 Point3D 类型的参数,并返回一个布尔类型的结果。
输出如下:
```
p1: (1,2,3)
p2: (4,5,6)
p2 is farther from the origin than p1
```
相关推荐
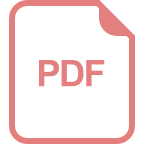
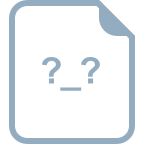
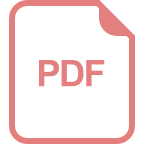














