实现迪杰斯特拉最短路径算法
时间: 2023-10-18 16:04:42 浏览: 90
Dijkstra算法是一种单源最短路径算法,用于计算一个节点到其他所有节点的最短路径。
下面是一个示例实现:
```python
import heapq
def dijkstra(graph, start):
# 初始化距离字典
dist = {node: float('inf') for node in graph}
dist[start] = 0
# 初始化堆
heap = [(0, start)]
while heap:
# 弹出堆中最小距离的节点
(distance, node) = heapq.heappop(heap)
# 更新周围节点的距离
for neighbor, weight in graph[node].items():
new_distance = dist[node] + weight
if new_distance < dist[neighbor]:
dist[neighbor] = new_distance
heapq.heappush(heap, (new_distance, neighbor))
return dist
```
其中,`graph` 是一个字典,键是节点,值是另一个字典,表示与该节点直接相邻的节点和它们之间的距离。`start` 是起点。
该函数返回一个字典,键是节点,值是从起点到该节点的最短距离。如果某个节点无法到达,则距离为无穷大。
阅读全文
相关推荐
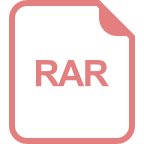








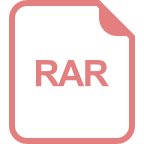




