feistel加解密算法
时间: 2023-07-22 22:54:52 浏览: 49
Feistel加解密算法是一种对称密钥加密算法,它采用了迭代式的加密方式。该算法的基本思想是将明文分成两个部分,然后分别进行多轮的加密和解密,最后再将两个部分合并成密文。在每一轮加密和解密中,算法都会根据密钥和轮数来生成一个子密钥,然后将其中一个部分作为输入,经过一系列的变换后,与另一个部分进行异或运算,得到新的部分,作为下一轮的输入。最后一轮得到的两个部分交换位置,然后合并成密文。解密过程与加密过程类似,只是子密钥的使用顺序相反。经过多轮迭代后,可以得到高度保密的加密结果。Feistel加解密算法被广泛应用于各种加密场景,如DES、AES等加密算法都采用了这种迭代式加密方式。
相关问题
c语言实现Feistel加解密算法
Feistel加解密算法是一种基于轮函数的分组密码算法,其核心思想是将明文分成左右两部分,每轮交替进行一系列运算,最终得到密文。下面是一个简单的C语言实现。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BLOCK_SIZE 8
#define ROUNDS 16
#define KEY_SIZE 10
typedef unsigned char byte;
const byte S_BOX[4][16] = {
{0x1, 0x0, 0x3, 0x2, 0x5, 0x4, 0x7, 0x6, 0x9, 0x8, 0xb, 0xa, 0xd, 0xc, 0xf, 0xe},
{0xe, 0xf, 0xc, 0xd, 0xa, 0xb, 0x8, 0x9, 0x6, 0x7, 0x4, 0x5, 0x2, 0x3, 0x0, 0x1},
{0x7, 0x6, 0x5, 0x4, 0x3, 0x2, 0x1, 0x0, 0xf, 0xe, 0xd, 0xc, 0xb, 0xa, 0x9, 0x8},
{0x8, 0x9, 0xa, 0xb, 0xc, 0xd, 0xe, 0xf, 0x0, 0x1, 0x2, 0x3, 0x4, 0x5, 0x6, 0x7}
};
const byte P_BOX[32] = {
16, 7, 20, 21,
29, 12, 28, 17,
1, 15, 23, 26,
5, 18, 31, 10,
2, 8, 24, 14,
32, 27, 3, 9,
19, 13, 30, 6,
22, 11, 4, 25
};
void feistel_encrypt(byte *block, byte *key) {
byte left[BLOCK_SIZE / 2], right[BLOCK_SIZE / 2];
memcpy(left, block, BLOCK_SIZE / 2);
memcpy(right, block + BLOCK_SIZE / 2, BLOCK_SIZE / 2);
for (int i = 0; i < ROUNDS; i++) {
byte temp[BLOCK_SIZE / 2];
memcpy(temp, left, BLOCK_SIZE / 2);
memcpy(left, right, BLOCK_SIZE / 2);
byte f_result[BLOCK_SIZE / 2];
for (int j = 0; j < BLOCK_SIZE / 2; j++) {
f_result[j] = S_BOX[j % 4][right[j] ^ key[j % KEY_SIZE]];
}
for (int j = 0; j < BLOCK_SIZE / 2; j++) {
right[j] = temp[j] ^ f_result[j];
}
}
memcpy(block, right, BLOCK_SIZE / 2);
memcpy(block + BLOCK_SIZE / 2, left, BLOCK_SIZE / 2);
}
void feistel_decrypt(byte *block, byte *key) {
byte left[BLOCK_SIZE / 2], right[BLOCK_SIZE / 2];
memcpy(left, block, BLOCK_SIZE / 2);
memcpy(right, block + BLOCK_SIZE / 2, BLOCK_SIZE / 2);
for (int i = ROUNDS - 1; i >= 0; i--) {
byte temp[BLOCK_SIZE / 2];
memcpy(temp, right, BLOCK_SIZE / 2);
memcpy(right, left, BLOCK_SIZE / 2);
byte f_result[BLOCK_SIZE / 2];
for (int j = 0; j < BLOCK_SIZE / 2; j++) {
f_result[j] = S_BOX[j % 4][left[j] ^ key[j % KEY_SIZE]];
}
for (int j = 0; j < BLOCK_SIZE / 2; j++) {
left[j] = temp[j] ^ f_result[j];
}
}
memcpy(block, left, BLOCK_SIZE / 2);
memcpy(block + BLOCK_SIZE / 2, right, BLOCK_SIZE / 2);
}
int main() {
byte plaintext[] = {0x48, 0x65, 0x6c, 0x6c, 0x6f, 0x2c, 0x20, 0x57};
byte key[] = {0x01, 0x23, 0x45, 0x67, 0x89, 0xab, 0xcd, 0xef, 0xfe, 0xdc};
byte ciphertext[BLOCK_SIZE];
printf("Plaintext: ");
for (int i = 0; i < BLOCK_SIZE; i++) {
printf("%02x ", plaintext[i]);
}
printf("\n");
feistel_encrypt(plaintext, key);
printf("Ciphertext: ");
for (int i = 0; i < BLOCK_SIZE; i++) {
printf("%02x ", plaintext[i]);
}
printf("\n");
feistel_decrypt(plaintext, key);
printf("Plaintext: ");
for (int i = 0; i < BLOCK_SIZE; i++) {
printf("%02x ", plaintext[i]);
}
printf("\n");
return 0;
}
```
在这个实现中,我们使用了8字节的明文和密文块,10字节的密钥。S_BOX是四个4x4的S盒,而P_BOX是一个32位的置换表。在加密和解密过程中,我们将明文块分成左右两部分,每轮交替进行一系列运算,最终得到密文或明文。在每轮中,我们首先将左半部分备份,将右半部分作为轮函数的输入,然后通过S盒和密钥生成一个4字节的输出,再通过P盒进行置换,最后与原来的左半部分异或得到新的右半部分。在解密过程中,我们只需要将加密过程中的操作顺序反转即可。
注意,这个实现中只是一个简单的Feistel加解密算法,不能用于实际的加密任务。如果你需要一个更安全的加密算法,请使用标准的密码库,如OpenSSL。
c++实现Feistel加解密
Feistel加密算法是一种常见的对称加密算法,其基本思想是将明文分成两部分,然后通过一定的加密函数和轮数来进行加密,最后将加密后的两部分再进行合并,得到最终的密文。解密时,只需要将加密过程中的加密函数反向使用即可。
以下是一个使用C语言实现的Feistel加解密算法示例:
```c
#include <stdio.h>
#include <string.h>
// 定义加密函数
int feistel_encrypt(int left, int right, int key) {
int tmp = left;
left = right ^ (left ^ key);
right = tmp;
return (left << 16) | right;
}
// 定义解密函数
int feistel_decrypt(int left, int right, int key) {
int tmp = right;
right = left ^ (right ^ key);
left = tmp;
return (left << 16) | right;
}
// 定义加密算法
void encrypt(char *plaintext, int *ciphertext, int key, int rounds) {
int len = strlen(plaintext);
int i, j;
for (i = 0; i < len; i += 4) {
int left = 0, right = 0;
for (j = 0; j < 4; j++) {
if (i + j < len) {
left <<= 8;
left |= plaintext[i + j];
}
}
for (j = 0; j < rounds; j++) {
int tmp = left;
left = feistel_encrypt(left, right, key);
right = tmp;
}
ciphertext[i / 4] = left | (right << 16);
}
}
// 定义解密算法
void decrypt(int *ciphertext, char *plaintext, int key, int rounds) {
int len = strlen(plaintext);
int i, j;
for (i = 0; i < len; i += 4) {
int left = ciphertext[i / 4] & 0xFFFF;
int right = ciphertext[i / 4] >> 16;
for (j = 0; j < rounds; j++) {
int tmp = left;
left = feistel_decrypt(left, right, key);
right = tmp;
}
for (j = 0; j < 4; j++) {
if (i + j < len) {
plaintext[i + j] = (left >> ((3 - j) * 8)) & 0xFF;
}
}
}
plaintext[len] = '\0';
}
int main() {
char plaintext[100] = "hello world";
int ciphertext[100];
int key = 0x12345678;
int rounds = 16;
encrypt(plaintext, ciphertext, key, rounds);
printf("加密后的密文:");
int i;
for (i = 0; i < strlen(plaintext); i += 4) {
printf("%08X ", ciphertext[i / 4]);
}
printf("\n");
char decrypted[100];
decrypt(ciphertext, decrypted, key, rounds);
printf("解密后的明文:%s\n", decrypted);
return 0;
}
```
上述代码中,`feistel_encrypt`函数实现了Feistel算法的加密过程,`feistel_decrypt`函数实现了Feistel算法的解密过程。`encrypt`函数实现了整个加密过程,其中将明文分为两部分,然后通过多轮Feistel算法进行加密,最后将加密后的两部分再进行合并得到密文。`decrypt`函数则实现了整个解密过程,其中将密文分为两部分,然后通过多轮Feistel算法进行解密,最后将解密后的两部分再进行合并得到明文。
相关推荐
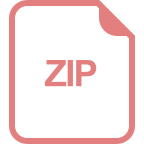
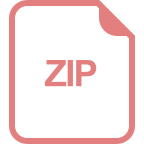












