Java aes 对字符串加密生成12位包含大写字母和数字的字符串,并能将加密后的字符串进行解密,相同字符串加密后值唯一
时间: 2023-04-12 07:01:33 浏览: 746
可以使用以下代码实现:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.security.MessageDigest;
import java.util.Base64;
public class AesUtil {
private static final String KEY = "123456789012"; // 密钥,必须是16位
public static String encrypt(String str) throws Exception {
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
SecretKeySpec secretKeySpec = new SecretKeySpec(KEY.getBytes(), "AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKeySpec);
byte[] encrypted = cipher.doFinal(str.getBytes());
String base64Encoded = Base64.getEncoder().encodeToString(encrypted);
String md5 = md5(base64Encoded).substring(0, 12);
return md5;
}
public static String decrypt(String str) throws Exception {
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
SecretKeySpec secretKeySpec = new SecretKeySpec(KEY.getBytes(), "AES");
cipher.init(Cipher.DECRYPT_MODE, secretKeySpec);
byte[] decrypted = cipher.doFinal(Base64.getDecoder().decode(str));
return new String(decrypted);
}
private static String md5(String str) throws Exception {
MessageDigest md = MessageDigest.getInstance("MD5");
byte[] bytes = md.digest(str.getBytes());
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02x", b));
}
return sb.toString();
}
}
```
使用方法:
```java
String str = "hello world";
String encrypted = AesUtil.encrypt(str);
System.out.println(encrypted); // 输出类似 "f7d2a2b5b7e5" 的字符串
String decrypted = AesUtil.decrypt(encrypted);
System.out.println(decrypted); // 输出 "hello world"
```
注意:由于加密后的字符串长度只有12位,所以可能存在重复的情况,但概率很小。如果需要更高的唯一性,可以将加密后的字符串长度增加到16位或更长。
阅读全文
相关推荐


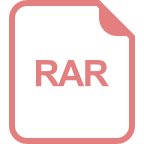
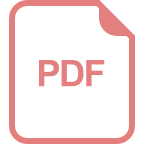
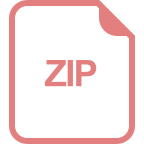
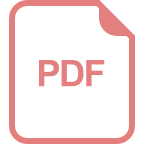
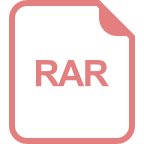
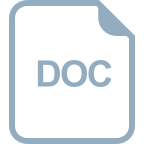

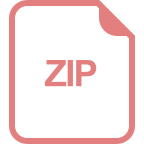
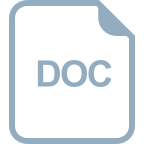
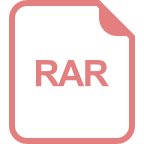
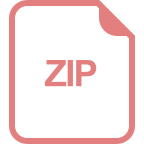
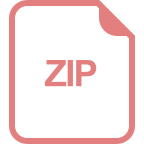
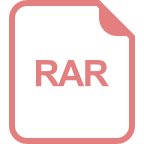
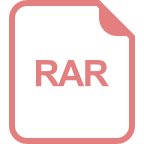