在c++中,不实用strcmp函数实现字符串的比较大小
时间: 2024-02-06 14:09:52 浏览: 30
可以使用C++中的运算符重载来实现字符串的比较大小。具体实现方法如下:
```c++
#include <iostream>
#include <cstring>
using namespace std;
class String {
public:
String(const char* str) {
m_data = new char[strlen(str) + 1];
strcpy(m_data, str);
}
~String() {
delete[] m_data;
}
bool operator<(const String& other) const {
return strcmp(m_data, other.m_data) < 0;
}
bool operator>(const String& other) const {
return strcmp(m_data, other.m_data) > 0;
}
private:
char* m_data;
};
int main() {
String s1("hello");
String s2("world");
if (s1 < s2) {
cout << "s1 < s2" << endl;
} else if (s1 > s2) {
cout << "s1 > s2" << endl;
} else {
cout << "s1 == s2" << endl;
}
return 0;
}
```
在上述代码中,我们定义了一个String类,重载了小于号和大于号运算符,来实现字符串的比较大小。在比较时,我们使用了strcmp函数来比较两个字符串的大小关系。在实际应用中,我们可以通过运算符重载来方便地比较字符串的大小。
相关推荐
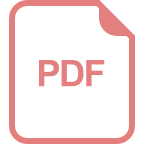














