在c++中,不使用strcmp函数实现字符串的比较大小
时间: 2024-02-06 07:09:53 浏览: 25
在 C++ 中,可以使用运算符重载的方式实现字符串的比较大小,以下是一个示例:
```c++
#include <iostream>
#include <cstring>
using namespace std;
class MyString {
private:
char *str;
int len;
public:
MyString(const char *s = "") {
len = strlen(s);
str = new char[len + 1];
strcpy(str, s);
}
~MyString() {
delete[] str;
}
bool operator<(const MyString &other) const {
return strcmp(str, other.str) < 0;
}
bool operator>(const MyString &other) const {
return strcmp(str, other.str) > 0;
}
bool operator==(const MyString &other) const {
return strcmp(str, other.str) == 0;
}
};
int main() {
MyString s1("abc");
MyString s2("def");
if (s1 < s2) {
cout << "s1 < s2" << endl;
} else if (s1 > s2) {
cout << "s1 > s2" << endl;
} else {
cout << "s1 == s2" << endl;
}
return 0;
}
```
在这个示例中,我们定义了一个 MyString 类,其中重载了 `<`, `>` 和 `==` 运算符用于字符串的比较大小。这样,我们就可以直接使用运算符进行比较,而不需要调用 strcmp 函数。
相关推荐
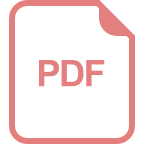














