在c++中,利用字符指针比较字符串s1、s2的大小
时间: 2024-02-03 15:14:17 浏览: 35
可以使用C++标准库中的字符串比较函数strcmp()来比较两个字符串s1和s2的大小。具体代码如下:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char* s1 = "hello";
char* s2 = "world";
int result = strcmp(s1, s2);
if (result < 0) {
cout << "s1 is less than s2" << endl;
} else if (result > 0) {
cout << "s1 is greater than s2" << endl;
} else {
cout << "s1 is equal to s2" << endl;
}
return 0;
}
```
在这个例子中,我们定义了两个字符指针s1和s2,并使用strcmp()函数比较它们的大小。如果s1小于s2,则strcmp()返回一个负数;如果s1大于s2,则返回一个正数;如果s1等于s2,则返回0。根据返回值的不同,我们可以输出相应的结果。
相关问题
请输出一个利用指针比较字符串的s1
以下是一个利用指针比较两个字符串s1和s2的示例程序:
```c++
#include <iostream>
using namespace std;
int main() {
char s1[] = "Hello, world!";
char s2[] = "hello, world!";
char* p1 = s1;
char* p2 = s2;
while (*p1 != '\0' && *p2 != '\0') {
if (*p1 != *p2) {
cout << "Strings are not equal" << endl;
return 0;
}
p1++;
p2++;
}
if (*p1 == '\0' && *p2 == '\0') {
cout << "Strings are equal" << endl;
} else {
cout << "Strings are not equal" << endl;
}
return 0;
}
```
该程序将两个字符串s1和s2赋值给指向字符数组的指针p1和p2。然后,进入一个while循环,只要p1和p2指向的字符都不为空,就比较它们指向的字符是否相同。如果相同,就将指针向后移动一个位置,继续比较下一个字符。如果不相同,就说明两个字符串不相等,跳出循环并输出结果。最后,如果两个指针都指向字符串的末尾(即字符'\0'),则说明两个字符串相等,输出结果。
C++指针连接字符串
C++指针连接字符串是指使用指针将两个字符串连接成一个字符串。在C++中,可以使用指针和循环来实现字符串的连接。常见的方法有三种,分别是方法一、方法二和方法三。
方法一:使用指针和循环实现字符串的连接。首先定义两个字符数组s1和s2,然后使用指针和循环将s2中的字符逐个复制到s1的末尾,最后在s1的末尾添加一个空字符'\0'。
方法二:使用指针和循环实现字符串的连接。首先定义两个字符数组s1和s2,然后使用指针和循环将s2中的字符逐个复制到s1的末尾,最后在s1的末尾添加一个空字符'\0'。与方法一不同的是,方法二使用了两个while循环来实现字符串的连接。
方法三:使用指针和循环实现字符串的连接。首先定义两个字符数组s1和s2,然后使用指针和循环将s2中的字符逐个复制到s1的末尾,最后在s1的末尾添加一个空字符'\0'。与方法一和方法二不同的是,方法三使用了一个while循环和一个for循环来实现字符串的连接。
相关推荐
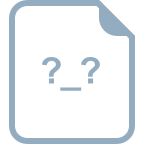
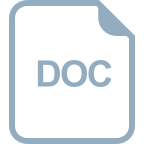












