C语言编写 UDS服务 1101的代码
时间: 2024-02-17 10:02:38 浏览: 210
UDS (Unified Diagnostic Services) 是一种面向汽车电子控制系统的诊断协议。其中,服务 0x1101 是请求 ECU 的诊断标识符。下面是一个简单的 C 语言程序,用于实现 UDS 服务 0x1101:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <sys/un.h>
#define SOCKET_PATH "/tmp/uds_socket" // UDS Socket 路径
#define SERVICE_ID 0x1101 // UDS 服务 ID
int main() {
int sock_fd, conn_fd;
struct sockaddr_un addr;
char buf[1024];
int len;
// 创建 Socket
sock_fd = socket(AF_UNIX, SOCK_STREAM, 0);
if (sock_fd < 0) {
perror("socket");
exit(1);
}
// 绑定 Socket
memset(&addr, 0, sizeof(addr));
addr.sun_family = AF_UNIX;
strncpy(addr.sun_path, SOCKET_PATH, sizeof(addr.sun_path) - 1);
if (bind(sock_fd, (struct sockaddr *)&addr, sizeof(addr)) < 0) {
perror("bind");
exit(1);
}
// 监听 Socket
if (listen(sock_fd, 5) < 0) {
perror("listen");
exit(1);
}
// 接受连接请求
conn_fd = accept(sock_fd, NULL, NULL);
if (conn_fd < 0) {
perror("accept");
exit(1);
}
// 读取请求数据
len = read(conn_fd, buf, sizeof(buf));
if (len < 0) {
perror("read");
exit(1);
}
// 处理请求
if (len >= 2 && buf[0] == SERVICE_ID && buf[1] == 0x00) {
// 请求 ECU 诊断标识符
char response[] = { SERVICE_ID, 0x02, 0x11, 0x22 }; // 返回诊断标识符 0x1122
write(conn_fd, response, sizeof(response));
} else {
// 其他未实现的服务
char response[] = { 0x7F, SERVICE_ID, 0x12, 0x34 }; // 返回未实现服务响应码
write(conn_fd, response, sizeof(response));
}
// 关闭连接
close(conn_fd);
return 0;
}
```
在上述代码中,我们使用 Unix 域套接字 (AF_UNIX) 来实现 UDS 服务。程序先创建 Socket 并绑定到指定的路径,然后监听 Socket 并接受连接请求。当有客户端连接到 Socket 后,程序从连接中读取请求数据,并判断是否是请求 ECU 诊断标识符的服务 (0x1101)。如果是,则返回诊断标识符 0x1122,否则返回未实现服务响应码。最后,程序关闭连接并退出。
阅读全文
相关推荐
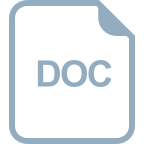
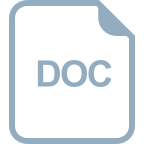
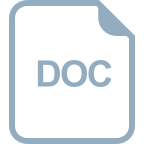



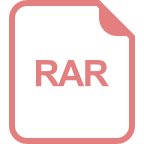
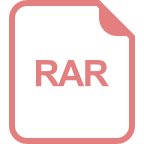
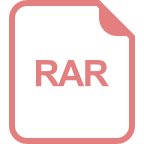
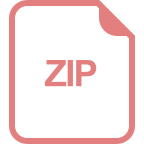
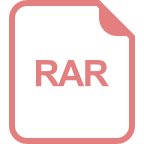
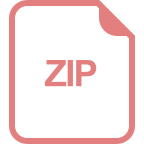
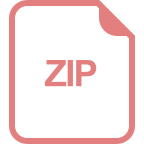
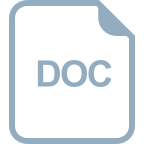
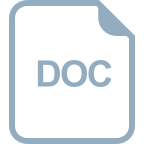
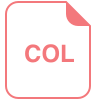


