用HTML+CSS+JS写一个炫酷的时钟显示效果
时间: 2024-01-13 08:04:31 浏览: 131
好的,下面是一份使用 HTML+CSS+JS 实现炫酷时钟的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>Cool Clock</title>
<style type="text/css">
body {
background-color: #333;
}
.clock {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
border-radius: 50%;
background-color: #fff;
box-shadow: 0 0 20px rgba(0, 0, 0, 0.1);
width: 300px;
height: 300px;
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
}
.clock .hour,
.clock .minute,
.clock .second {
position: relative;
width: 70%;
height: 70%;
margin: auto;
border-radius: 50%;
background-color: #333;
display: flex;
align-items: center;
justify-content: center;
font-size: 2rem;
color: #fff;
}
.clock .hour:before,
.clock .minute:before,
.clock .second:before {
content: "";
position: absolute;
top: 0;
left: 50%;
transform: translate(-50%, 0);
height: 50%;
width: 5px;
background-color: #fff;
border-radius: 5px;
}
.clock .hour {
z-index: 3;
}
.clock .hour:before {
height: 30%;
}
.clock .minute {
z-index: 2;
}
.clock .minute:before {
height: 40%;
}
.clock .second {
z-index: 1;
}
.clock .second:before {
height: 50%;
}
.clock .tick {
position: absolute;
top: 0;
left: 50%;
transform: translate(-50%, 0);
height: 10%;
width: 5px;
background-color: #fff;
border-radius: 5px;
transform-origin: center 90%;
animation: tick 1s linear infinite;
}
.clock .tick:nth-child(5n+1) {
height: 20%;
}
.clock .tick:nth-child(10n+1) {
height: 30%;
}
.clock .tick:nth-child(15n+1) {
height: 40%;
}
.clock .tick:nth-child(20n+1) {
height: 50%;
}
@keyframes tick {
from {
transform: rotate(0deg);
}
to {
transform: rotate(360deg);
}
}
</style>
</head>
<body>
<div class="clock">
<div class="hour"></div>
<div class="minute"></div>
<div class="second"></div>
<div class="tick"></div>
<div class="tick"></div>
<div class="tick"></div>
<div class="tick"></div>
<div class="tick"></div>
<div class="tick"></div>
<div class="tick"></div>
<div class="tick"></div>
<div class="tick"></div>
<div class="tick"></div>
</div>
<script type="text/javascript">
function updateClock() {
var now = new Date();
var hour = now.getHours();
var minute = now.getMinutes();
var second = now.getSeconds();
var hourHand = document.querySelector(".hour");
var minuteHand = document.querySelector(".minute");
var secondHand = document.querySelector(".second");
var hourAngle = (hour % 12) / 12 * 360 + minute / 60 * 30;
var minuteAngle = minute / 60 * 360;
var secondAngle = second / 60 * 360;
hourHand.style.transform = "rotate(" + hourAngle + "deg)";
minuteHand.style.transform = "rotate(" + minuteAngle + "deg)";
secondHand.style.transform = "rotate(" + secondAngle + "deg)";
}
setInterval(updateClock, 1000);
</script>
</body>
</html>
```
这份代码实现了一个圆形的时钟,时钟的样式可以通过 CSS 进行自定义。时钟的指针是通过 CSS 的 `transform: rotate()` 属性来控制旋转角度的,指针的角度是根据当前时间计算出来的。时钟上的刻度线是通过 CSS 的 `animation` 属性来实现的,用 `transform-origin` 控制旋转中心,用 `@keyframes` 定义动画。
阅读全文
相关推荐
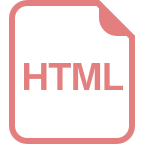
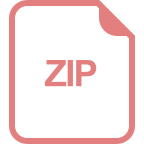
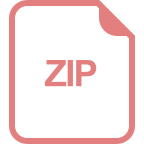
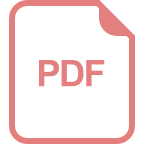
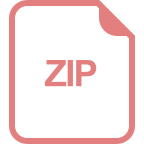
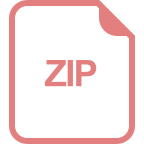
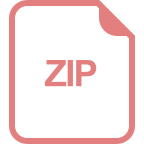
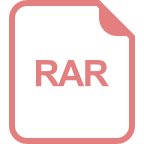
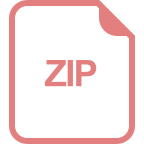
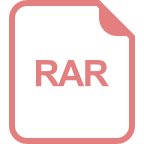
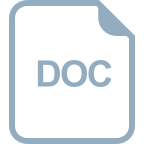
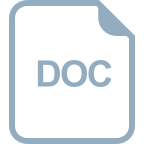
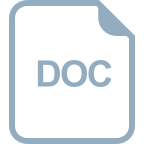

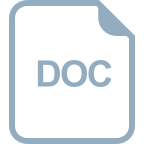