使用pytorch模型将深度信息融合到图片中
时间: 2024-05-08 11:15:47 浏览: 88
要将深度信息融合到图片中,可以使用下面的步骤:
1. 加载图片和深度信息。
```python
import cv2
import numpy as np
# 加载图片和深度信息
img = cv2.imread("image.jpg")
depth = cv2.imread("depth.png", cv2.IMREAD_GRAYSCALE)
```
2. 对深度信息进行预处理,使其与图片大小相同。
```python
# 对深度信息进行预处理,使其与图片大小相同
depth = cv2.resize(depth, (img.shape[1], img.shape[0]))
depth = np.expand_dims(depth, axis=2)
depth = depth.astype(np.float32) / 255.0
```
3. 将图片和深度信息转换为PyTorch张量。
```python
import torch
# 将图片和深度信息转换为PyTorch张量
img_tensor = torch.from_numpy(img.transpose((2, 0, 1))).float().unsqueeze(0)
depth_tensor = torch.from_numpy(depth.transpose((2, 0, 1))).float().unsqueeze(0)
```
4. 定义模型,将深度信息融合到图片中。
```python
import torch.nn as nn
# 定义模型,将深度信息融合到图片中
class DepthFusion(nn.Module):
def __init__(self):
super(DepthFusion, self).__init__()
self.conv1 = nn.Conv2d(4, 32, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(32, 32, kernel_size=3, stride=1, padding=1)
self.conv3 = nn.Conv2d(32, 3, kernel_size=3, stride=1, padding=1)
self.relu = nn.ReLU()
def forward(self, x):
x = self.relu(self.conv1(x))
x = self.relu(self.conv2(x))
x = self.conv3(x)
return x
model = DepthFusion()
```
5. 将图片和深度信息输入模型,并得到融合后的结果。
```python
# 将图片和深度信息输入模型,并得到融合后的结果
input_tensor = torch.cat((img_tensor, depth_tensor), dim=1)
output_tensor = model(input_tensor)
# 将结果转换为NumPy数组,并显示
output = output_tensor.detach().numpy()[0].transpose((1, 2, 0))
cv2.imshow("Output", output)
cv2.waitKey(0)
```
这样,就可以将深度信息融合到图片中了。
阅读全文
相关推荐


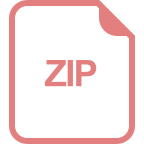
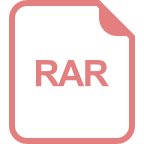
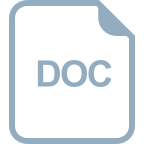
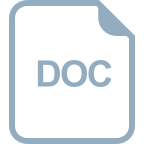
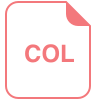
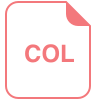
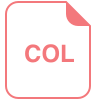
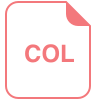
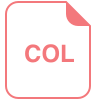




