使用pytorch 实现将图片信息和深度信息融合在一起
时间: 2023-12-09 21:07:01 浏览: 37
以下是一个简单的示例,展示如何将图像信息和深度信息融合在一起。
首先,我们需要导入PyTorch库和一些必要的模块。
```
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch.utils.data import Dataset, DataLoader
from torchvision import transforms
from PIL import Image
import numpy as np
```
接下来,我们需要定义一个数据集,其中包含图像和深度图像。
```
class MyDataset(Dataset):
def __init__(self, img_dir, depth_dir):
self.img_dir = img_dir
self.depth_dir = depth_dir
self.transform = transforms.Compose([
transforms.Resize((256, 256)),
transforms.ToTensor()
])
def __len__(self):
return len(os.listdir(self.img_dir))
def __getitem__(self, idx):
img_path = os.path.join(self.img_dir, f"img_{idx}.jpg")
depth_path = os.path.join(self.depth_dir, f"depth_{idx}.npy")
img = Image.open(img_path).convert('RGB')
img = self.transform(img)
depth = np.load(depth_path)
depth = torch.from_numpy(depth).unsqueeze(0)
return img, depth
```
在这个数据集中,我们假设有一些图像和对应的深度图像。我们将它们存储在两个不同的文件夹中,并通过MyDataset类加载它们。
接下来,我们需要定义一个模型,它将图像和深度信息融合在一起。在这个简单的示例中,我们使用一个简单的卷积神经网络模型,它将图像和深度图像连接在一起。
```
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.conv1 = nn.Conv2d(4, 16, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(16, 32, kernel_size=3, stride=1, padding=1)
self.conv3 = nn.Conv2d(32, 64, kernel_size=3, stride=1, padding=1)
self.conv4 = nn.Conv2d(64, 128, kernel_size=3, stride=1, padding=1)
self.fc1 = nn.Linear(128 * 16 * 16, 256)
self.fc2 = nn.Linear(256, 2)
def forward(self, x, d):
x = torch.cat((x, d), dim=1)
x = F.relu(self.conv1(x))
x = F.max_pool2d(x, 2)
x = F.relu(self.conv2(x))
x = F.max_pool2d(x, 2)
x = F.relu(self.conv3(x))
x = F.max_pool2d(x, 2)
x = F.relu(self.conv4(x))
x = F.max_pool2d(x, 2)
x = x.view(-1, 128 * 16 * 16)
x = F.relu(self.fc1(x))
x = self.fc2(x)
return x
```
在这个模型中,我们将图像和深度信息连接在一起,并通过一些卷积层和全连接层进行处理。最后,我们输出一个包含两个元素的向量,这些元素代表某些类别的概率。
接下来,我们需要训练模型。
```
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
img_dir = "path/to/image/folder/"
depth_dir = "path/to/depth/folder/"
dataset = MyDataset(img_dir, depth_dir)
dataloader = DataLoader(dataset, batch_size=32, shuffle=True)
model = MyModel().to(device)
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
for epoch in range(10):
for i, (images, depths) in enumerate(dataloader):
images = images.to(device)
depths = depths.to(device)
labels = torch.zeros(images.shape[0], dtype=torch.long).to(device)
optimizer.zero_grad()
outputs = model(images, depths)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
if (i+1) % 100 == 0:
print(f"Epoch [{epoch+1}/{10}], Step [{i+1}/{len(dataloader)}], Loss: {loss.item():.4f}")
```
在这个训练过程中,我们将数据加载到设备中,并使用Adam优化器进行反向传播。我们继续训练10个周期,并在每个周期结束时输出损失。
最后,我们可以使用模型来进行预测。
```
img_path = "path/to/test/image.jpg"
depth_path = "path/to/test/depth.npy"
img = Image.open(img_path).convert('RGB')
img = transforms.Resize((256, 256))(img)
img = transforms.ToTensor()(img)
depth = np.load(depth_path)
depth = torch.from_numpy(depth).unsqueeze(0)
model.eval()
with torch.no_grad():
output = model(img.unsqueeze(0), depth)
_, predicted = torch.max(output.data, 1)
print(predicted.item())
```
在这个预测中,我们将测试图像和深度图像加载到模型中,并输出预测的类别。
相关推荐
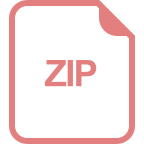
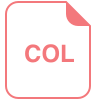













