使用STM32F103RCT6开发板,连接led二极管,控制二极管在白天关闭夜晚发光
时间: 2024-03-10 15:49:28 浏览: 72
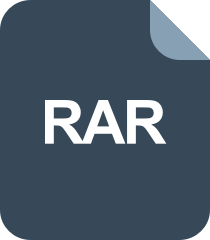
控制二极管发光
要实现这个功能,你需要使用光敏电阻或光敏二极管来检测环境光线强度,然后通过控制IO口输出高低电平来控制LED灯的亮灭。
具体实现步骤如下:
1. 将光敏电阻或光敏二极管接入开发板的ADC输入引脚。
2. 在开发板上搭建一个简单的电路,将LED灯连接到开发板的IO口输出引脚。
3. 编写程序,初始化ADC模块和IO口,配置ADC采样参数并启动采样。
4. 在程序中加入一个循环,不断读取ADC的采样值,并根据采样值判断当前的光线强度。
5. 根据光线强度控制IO口输出高低电平,从而控制LED的亮灭。
下面是一个简单的代码示例:
```c
#include "stm32f10x.h"
#define LED_PIN GPIO_Pin_0
#define LED_PORT GPIOA
void ADC_Configuration(void)
{
ADC_InitTypeDef ADC_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1 | RCC_APB2Periph_GPIOA, ENABLE);
// Configure ADC input pin
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_1;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AIN;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Configure ADC
ADC_InitStructure.ADC_Mode = ADC_Mode_Independent;
ADC_InitStructure.ADC_ScanConvMode = DISABLE;
ADC_InitStructure.ADC_ContinuousConvMode = ENABLE;
ADC_InitStructure.ADC_ExternalTrigConv = ADC_ExternalTrigConv_None;
ADC_InitStructure.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStructure.ADC_NbrOfChannel = 1;
ADC_Init(ADC1, &ADC_InitStructure);
// Enable ADC
ADC_Cmd(ADC1, ENABLE);
// Start ADC calibration
ADC_ResetCalibration(ADC1);
while (ADC_GetResetCalibrationStatus(ADC1))
;
ADC_StartCalibration(ADC1);
while (ADC_GetCalibrationStatus(ADC1))
;
// Start ADC conversion
ADC_SoftwareStartConvCmd(ADC1, ENABLE);
}
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// Configure LED pin
GPIO_InitStructure.GPIO_Pin = LED_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_PORT, &GPIO_InitStructure);
}
int main(void)
{
ADC_Configuration();
GPIO_Configuration();
while (1)
{
// Read ADC value
uint16_t adc_value = ADC_GetConversionValue(ADC1);
// Determine ambient light level
if (adc_value > 2048)
{
GPIO_SetBits(LED_PORT, LED_PIN); // Turn off LED
}
else
{
GPIO_ResetBits(LED_PORT, LED_PIN); // Turn on LED
}
}
}
```
这个代码示例中使用了ADC1的通道0来采集光敏电阻的数值,并根据数值大小来控制LED灯的亮灭。注意,这个示例中使用的是单通道的ADC采样方式,如果需要采集多个通道的数据,需要将ADC的扫描模式设置为ENABLE,并在ADC的通道序列寄存器中配置需要采集的通道。
阅读全文
相关推荐
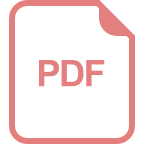
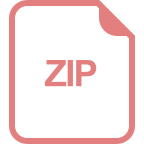
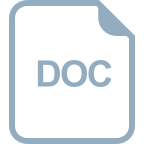
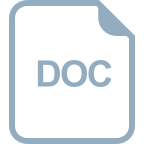
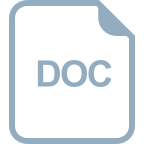
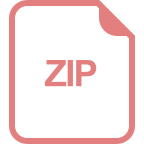
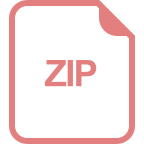
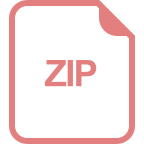
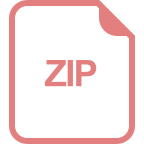
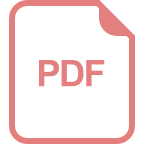
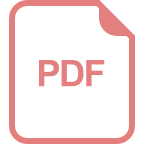
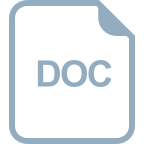
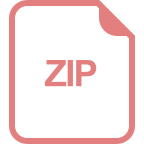
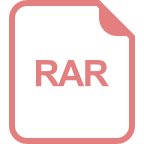
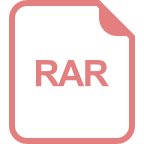