torch.randn(iter_adv.shape)
时间: 2023-11-01 15:41:10 浏览: 124
This line of code generates a tensor of random numbers from a standard normal distribution (mean=0, standard deviation=1) with the same shape as the tensor `iter_adv`. The `torch.randn` function is part of the PyTorch library and is commonly used for initializing weights or generating noise in deep learning models.
相关问题
torch.randn_like()
`torch.randn_like()` 是 PyTorch(一个开源的机器学习库)中的一个函数,它用于生成一个新的张量,其形状和数据类型与给定的输入张量 (`like` 参数) 完全相同,但元素值是随机从标准正态分布(mean=0, std=1)中抽取的。这个函数在深度学习中常用于初始化模型参数或创建随机噪声输入。
使用示例:
```python
import torch
# 假设你有一个张量 x
x = torch.tensor([[1, 2], [3, 4]])
# 使用 torch.randn_like(x) 生成与 x 同样大小和类型的随机张量
random_tensor = torch.randn_like(x)
```
def adversarial(x, model, loss_func, c=1e-4, kappa=0, num_iter=100, lr=0.01): """ Create adversarial examples using CW algorithm Args: - x: input image - model: the neural network model - loss_func: the loss function to use - c: the weight for the L2 regularization term (default=1e-4) - kappa: the confidence parameter (default=0) - num_iter: number of iterations for the algorithm (default=100) - lr: learning rate for the optimization (default=0.01) Returns: - x_adv: adversarial example """ x_adv = x.clone().detach().requires_grad_(True) for i in range(num_iter): output = model(x_adv) loss = loss_func(output, torch.tensor([kappa]), x, x_adv, c) model.zero_grad() loss.backward() with torch.no_grad(): x_adv += lr * x_adv.grad x_adv = torch.max(torch.min(x_adv, x + 0.35), x - 0.35) x_adv = torch.clamp(x_adv, 0, 1) x_adv.requires_grad_() return x_adv.detach()上述代码出现TypeError: ce_loss() takes 2 positional arguments but 5 were given错误,请改正
根据代码,`loss_func`应该是一个函数,且它的输入参数应该是模型的输出 `output`和目标标签 `target`。因此,需要修改 `loss_func` 函数的输入参数,将 `x`、`x_adv` 和 `c` 作为函数的参数,而不是在函数调用时传入。修改后的代码如下:
```
def adversarial(x, model, loss_func, c=1e-4, kappa=0, num_iter=100, lr=0.01):
"""Create adversarial examples using CW algorithm
Args:
- x: input image
- model: the neural network model
- loss_func: the loss function to use
- c: the weight for the L2 regularization term (default=1e-4)
- kappa: the confidence parameter (default=0)
- num_iter: number of iterations for the algorithm (default=100)
- lr: learning rate for the optimization (default=0.01)
Returns:
- x_adv: adversarial example
"""
x_adv = x.clone().detach().requires_grad_(True)
for i in range(num_iter):
output = model(x_adv)
loss = loss_func(output, kappa, target=x, adversary=x_adv, c=c)
model.zero_grad()
loss.backward()
with torch.no_grad():
x_adv += lr * x_adv.grad
x_adv = torch.max(torch.min(x_adv, x + 0.35), x - 0.35)
x_adv = torch.clamp(x_adv, 0, 1)
x_adv.requires_grad_()
return x_adv.detach()
```
阅读全文
相关推荐
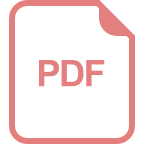
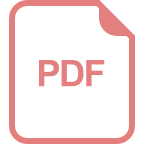













