用结构体,函数,指针,文件,写一个模拟发牌的c语言代码,一副牌发给四个人,没有大小王,牌的花色用ASCII表示
时间: 2023-06-01 16:02:25 浏览: 86
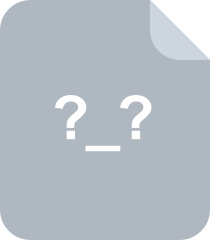
100 扑克牌自动发牌 C语言源代码文件
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define TOTAL_CARDS 52
#define NUM_OF_PLAYERS 4
typedef struct {
char suit; // 花色
int value; // 牌值
} Card;
void shuffle(Card *deck);
void deal(Card *deck, Card *players[]);
int main() {
Card deck[TOTAL_CARDS];
Card *players[NUM_OF_PLAYERS];
// 初始化牌堆
int count = 0;
for (char suit = 'A'; suit <= 'D'; suit++) {
for (int value = 1; value <= 13; value++) {
deck[count].suit = suit;
deck[count].value = value;
count++;
}
}
// 洗牌
shuffle(deck);
// 发牌
deal(deck, players);
// 输出每个玩家手中的牌
for (int i = 0; i < NUM_OF_PLAYERS; i++) {
printf("Player %d:\n", i+1);
for (int j = 0; j < TOTAL_CARDS/NUM_OF_PLAYERS; j++) {
printf("%c%d ", players[i][j].suit, players[i][j].value);
}
printf("\n");
}
// 释放内存
for (int i = 0; i < NUM_OF_PLAYERS; i++) {
free(players[i]);
}
return 0;
}
// 洗牌函数
void shuffle(Card *deck) {
srand(time(NULL));
for (int i = 0; i < TOTAL_CARDS; i++) {
int j = rand() % TOTAL_CARDS;
Card temp = deck[i];
deck[i] = deck[j];
deck[j] = temp;
}
}
// 发牌函数
void deal(Card *deck, Card *players[]) {
for (int i = 0; i < NUM_OF_PLAYERS; i++) {
players[i] = (Card*) malloc((TOTAL_CARDS/NUM_OF_PLAYERS) * sizeof(Card));
for (int j = 0; j < TOTAL_CARDS/NUM_OF_PLAYERS; j++) {
players[i][j] = deck[i*(TOTAL_CARDS/NUM_OF_PLAYERS)+j];
}
}
}
阅读全文
相关推荐
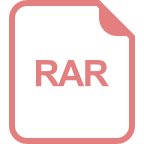


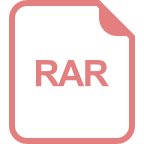
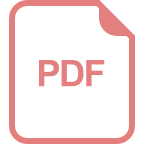
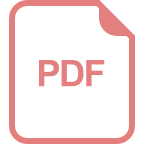
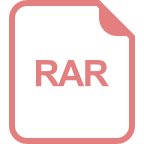
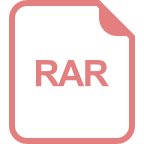
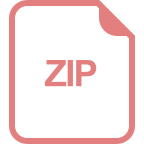
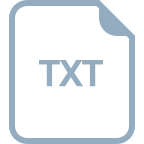
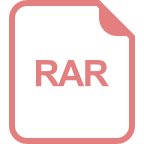
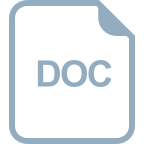
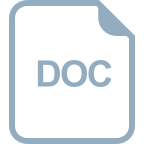
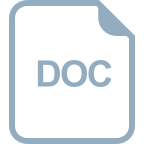
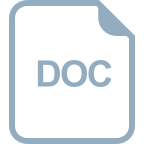
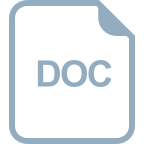
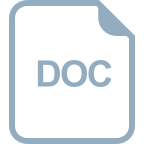