print("For a student with scores 45 and 85, we predict an admission probability of",prob[0])是什么意思
时间: 2023-06-26 15:04:44 浏览: 135
这行代码是在输出一个字符串和一个变量的值。其中,字符串内容为 "For a student with scores 45 and 85, we predict an admission probability of",而变量 prob[0] 则表示模型预测出的该名学生被录取的概率值。整行代码的作用是输出该名学生的成绩和被录取概率的预测结果。
相关问题
Compute the loss and gradients for a two layer fully connected neural network. Inputs: - X: Input data of shape (N, D). Each X[i] is a training sample. - y: Vector of training labels. y[i] is the label for X[i], and each y[i] is an integer in the range 0 <= y[i] < C. This parameter is optional; if it is not passed then we only return scores, and if it is passed then we instead return the loss and gradients. - reg: Regularization strength. Returns: If y is None, return a matrix scores of shape (N, C) where scores[i, c] is the score for class c on input X[i]. If y is not None, instead return a tuple of: - loss: Loss (data loss and regularization loss) for this batch of training samples. - grads: Dictionary mapping parameter names to gradients of those parameters with respect to the loss function; has the same keys as self.params.
To compute the loss and gradients for a two layer fully connected neural network, we need to perform forward and backward propagation.
Forward propagation:
1. Compute the scores for each class by multiplying the input data X with the weight matrix W1 and adding the bias term b1. Then apply ReLU activation function to the result.
2. Compute the scores for each class by multiplying the output of the first layer with the weight matrix W2 and adding the bias term b2.
The loss function for a multi-class classification problem is usually the cross-entropy loss.
Backward propagation:
1. Compute the gradient of the loss with respect to the scores of the second layer.
2. Compute the gradient of the loss with respect to the parameters of the second layer (W2 and b2).
3. Compute the gradient of the loss with respect to the output of the first layer.
4. Compute the gradient of the loss with respect to the scores of the first layer (taking into account the ReLU activation function).
5. Compute the gradient of the loss with respect to the parameters of the first layer (W1 and b1).
Finally, we add the regularization term to the loss and compute the gradients with respect to the regularization term as well.
Here's the code:
```python
def two_layer_fc(X, params, reg=0.0):
W1, b1, W2, b2 = params['W1'], params['b1'], params['W2'], params['b2']
N, D = X.shape
scores = None
# Forward pass
hidden_layer = np.maximum(0, np.dot(X, W1) + b1) # ReLU activation
scores = np.dot(hidden_layer, W2) + b2
# If y is not given, return scores
if y is None:
return scores
# Compute the loss and gradients
loss = None
grads = {}
# Compute the loss (data loss and regularization loss)
num_classes = W2.shape[1]
exp_scores = np.exp(scores)
probs = exp_scores / np.sum(exp_scores, axis=1, keepdims=True)
correct_logprobs = -np.log(probs[range(N),y])
data_loss = np.sum(correct_logprobs) / N
reg_loss = 0.5 * reg * (np.sum(W1*W1) + np.sum(W2*W2))
loss = data_loss + reg_loss
# Compute the gradients
dscores = probs
dscores[range(N),y] -= 1
dscores /= N
dW2 = np.dot(hidden_layer.T, dscores)
db2 = np.sum(dscores, axis=0, keepdims=True)
dhidden = np.dot(dscores, W2.T)
dhidden[hidden_layer <= 0] = 0
dW1 = np.dot(X.T, dhidden)
db1 = np.sum(dhidden, axis=0, keepdims=True)
# Add regularization gradient contribution
dW2 += reg * W2
dW1 += reg * W1
# Store gradients in dictionary
grads['W1'] = dW1
grads['b1'] = db1
grads['W2'] = dW2
grads['b2'] = db2
return loss, grads
```
There is a midterm.csv file about the grading result of a course's midterm exam. The first row presents the header. Then, each row presents a student's enrolled name and his or her score. Write a program to read the file and collect all the scores in a list (without header row!) Download the midterm.csv file. Classify those scores according to the criteria given by question 2 in Problem Set 1. Then, draw a histogram with plotly for ranks A, B, C, D, F
Sure! For reading and processing the CSV file, we can use the `csv` module in Python. Here's the code to read and collect all the scores in a list:
```python
import csv
scores = []
with open('midterm.csv', newline='') as csvfile:
reader = csv.reader(csvfile)
next(reader) # skip header row
for row in reader:
scores.append(int(row[1]))
print(scores)
```
This code will create a list `scores` containing all the numeric scores from the CSV file.
For the second part of the question, we need to classify the scores into A, B, C, D, and F categories. According to the criteria given in Problem Set 1, we can use the following classification:
- A: score >= 90
- B: 80 <= score < 90
- C: 70 <= score < 80
- D: 60 <= score < 70
- F: score < 60
Here's the code to classify the scores and count the number of scores in each category:
```python
counts = {'A': 0, 'B': 0, 'C': 0, 'D': 0, 'F': 0}
for score in scores:
if score >= 90:
counts['A'] += 1
elif score >= 80:
counts['B'] += 1
elif score >= 70:
counts['C'] += 1
elif score >= 60:
counts['D'] += 1
else:
counts['F'] += 1
print(counts)
```
This code will create a dictionary `counts` containing the number of scores in each category.
Finally, we can use the `plotly` library to create a histogram of the scores. Here's the complete code:
```python
import csv
import plotly.graph_objs as go
scores = []
with open('midterm.csv', newline='') as csvfile:
reader = csv.reader(csvfile)
next(reader) # skip header row
for row in reader:
scores.append(int(row[1]))
counts = {'A': 0, 'B': 0, 'C': 0, 'D': 0, 'F': 0}
for score in scores:
if score >= 90:
counts['A'] += 1
elif score >= 80:
counts['B'] += 1
elif score >= 70:
counts['C'] += 1
elif score >= 60:
counts['D'] += 1
else:
counts['F'] += 1
labels = ['A', 'B', 'C', 'D', 'F']
values = [counts[label] for label in labels]
fig = go.Figure([go.Bar(x=labels, y=values)])
fig.show()
```
This code will create a histogram of the scores, showing the number of scores in each category.
阅读全文
相关推荐
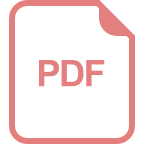
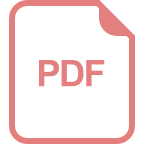
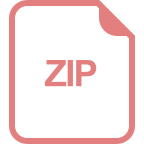
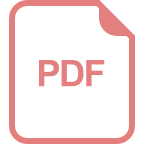
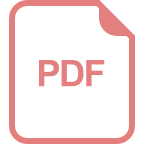
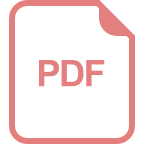








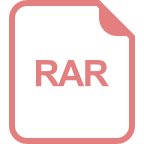
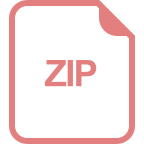