提供相关C语言中map的资料或示例代码
时间: 2024-05-06 13:21:06 浏览: 13
您好,以下是C语言中map的一个实现方式:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_HASH 1000000
typedef struct node {
char* key;
int value;
struct node* next;
} Node;
typedef struct {
Node* nodes[MAX_HASH];
} Map;
unsigned int hash(char* key) {
unsigned int hash = 0;
for (int i = 0; i < strlen(key); i++) {
hash = hash * 31 + key[i];
}
return hash % MAX_HASH;
}
Node* createNode(char* key, int value) {
Node* node = (Node*)malloc(sizeof(Node));
node->key = (char*)malloc(strlen(key) + 1);
strcpy(node->key, key);
node->value = value;
node->next = NULL;
return node;
}
void insert(Map* map, char* key, int value) {
unsigned int index = hash(key);
Node* node = map->nodes[index];
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
node->value = value;
return;
}
node = node->next;
}
node = createNode(key, value);
node->next = map->nodes[index];
map->nodes[index] = node;
}
int* find(Map* map, char* key) {
unsigned int index = hash(key);
Node* node = map->nodes[index];
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
return &(node->value);
}
node = node->next;
}
return NULL;
}
int main() {
Map map;
memset(&map, 0, sizeof(map));
insert(&map, "hello", 1);
insert(&map, "world", 2);
int* value = find(&map, "hello");
if (value != NULL) {
printf("hello: %d\n", *value);
} else {
printf("hello not found\n");
}
value = find(&map, "world");
if (value != NULL) {
printf("world: %d\n", *value);
} else {
printf("world not found\n");
}
return 0;
}
```
希望对您有用,您可以自己尝试运行代码看看效果。
相关推荐
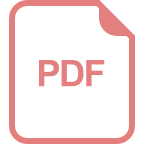
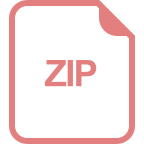
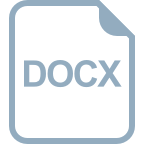














