编写一个程序,使用Runnable接口的方式创建两个线程,分别输出从0到10的数,每个线程之间延迟500毫秒,要求输出的结果如下所示: 线程一 : 0,1,2,3,4,5,6,7,8,9,10 线程二 : 0,1,2,3,4,5,6,7,8,9,10
时间: 2023-09-07 12:13:41 浏览: 107
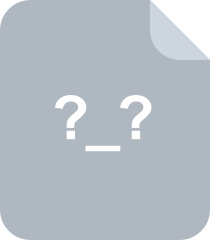
实现runnable接口创建线程
好的,以下是一个简单的Java程序,其中使用`Runnable`接口的方式创建了两个线程,分别输出从0到10的数,每个线程之间延迟500毫秒,并且在输出时显示是哪个线程输出的数。
```java
public class NumberPrinter {
public static void main(String[] args) {
Thread t1 = new Thread(new NumberRunnable("线程一"));
Thread t2 = new Thread(new NumberRunnable("线程二"));
t1.start();
t2.start();
}
private static class NumberRunnable implements Runnable {
private String threadName;
public NumberRunnable(String threadName) {
this.threadName = threadName;
}
@Override
public void run() {
for (int i = 0; i <= 10; i++) {
System.out.print(threadName + " : " + i);
if (i < 10) {
System.out.print(",");
}
try {
Thread.sleep(500);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println();
}
}
}
```
在这个程序中,我们定义了一个`NumberRunnable`类,它实现了`Runnable`接口,这样我们可以将它作为参数传递给`Thread`类的构造函数,从而创建一个新的线程。
在`NumberRunnable`类中,我们定义了一个成员变量`threadName`,用于存储当前线程的名称。在`run`方法中,我们使用一个`for`循环输出从0到10的数,并在每次输出时,打印出当前线程的名称和输出的数,并且在每个数之间加上逗号。然后,我们使用`Thread.sleep(500)`方法,让当前线程休眠500毫秒,以模拟两个线程之间的延迟。最后,我们在每个线程输出完所有数字后,打印一个换行符。
在`main`方法中,我们创建了两个线程,分别传入不同的`NumberRunnable`实例,然后调用它们的`start`方法,启动这两个线程。这样,两个线程就会同时执行,分别输出从0到10的数,并且在输出时显示是哪个线程输出的数。
阅读全文
相关推荐
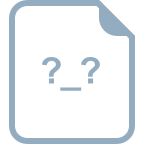



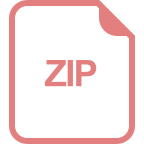
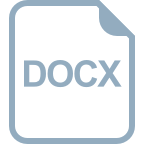
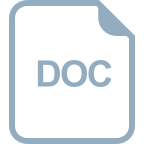
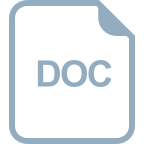
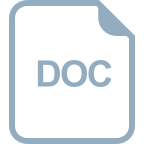
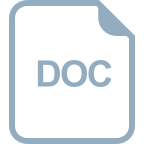
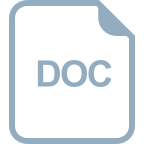
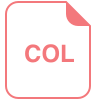
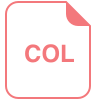
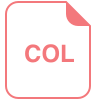
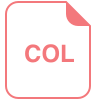
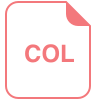
