用C语言使用深度优先求一个图的连通分量
时间: 2023-05-25 16:00:55 浏览: 56
要使用深度优先搜索算法来求一个图的联通分量,首先需要构建一个图的数据结构,并在其中存储节点之间的关系。这可以采用邻接表来实现,其中每个节点都与其连接的节点列表相关联。
以下是使用C语言实现深度优先搜索算法来求一个图的连通分量的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义邻接表结构体
struct AdjListNode {
int dest;
struct AdjListNode* next;
};
// 定义图结构体
struct Graph {
int V;
struct AdjListNode** adjList;
int* visited;
};
// 创建邻接表节点
struct AdjListNode* newAdjListNode(int dest) {
struct AdjListNode* newNode = (struct AdjListNode*) malloc(sizeof(struct AdjListNode));
newNode->dest = dest;
newNode->next = NULL;
return newNode;
}
// 创建图
struct Graph* createGraph(int V) {
struct Graph* graph = (struct Graph*) malloc(sizeof(struct Graph));
graph->V = V;
// 分配邻接表
graph->adjList = (struct AdjListNode**) malloc(V * sizeof(struct AdjListNode*));
graph->visited = (int*) malloc(V * sizeof(int));
// 初始化邻接表为空
for (int i = 0; i < V; ++i) {
graph->adjList[i] = NULL;
graph->visited[i] = 0;
}
return graph;
}
// 添加边到图中
void addEdge(struct Graph* graph, int src, int dest) {
struct AdjListNode* newNode = newAdjListNode(dest);
newNode->next = graph->adjList[src];
graph->adjList[src] = newNode;
newNode = newAdjListNode(src);
newNode->next = graph->adjList[dest];
graph->adjList[dest] = newNode;
}
// 深度优先搜索算法
void DFS(struct Graph* graph, int v) {
// 标记当前节点已访问
graph->visited[v] = 1;
printf("%d ", v);
// 遍历邻接表中的节点
struct AdjListNode* node = graph->adjList[v];
while (node != NULL) {
int adj = node->dest;
if (!graph->visited[adj]) {
DFS(graph, adj);
}
node = node->next;
}
}
// 执行深度优先搜索并输出连通分量
void getConnectedComponents(struct Graph* graph) {
for (int i = 0; i < graph->V; ++i) {
if (!graph->visited[i]) {
printf("Connected component %d: ", i);
DFS(graph, i);
printf("\n");
}
}
}
// 主函数
int main() {
int V = 5;
struct Graph* graph = createGraph(V);
addEdge(graph, 0, 1);
addEdge(graph, 1, 2);
addEdge(graph, 3, 4);
getConnectedComponents(graph);
return 0;
}
```
这段代码中,我们定义了一个用邻接表表示图的结构体,其中包含有向边的列表。我们还定义了一个图结构体,它由一个图大小变量组成,一个指向邻接表的指针数组以及一个标志是否已访问过该节点的数组。我们还定义了一个深度优先搜索函数(getConnectedComponents),它在迭代未访问过的节点时调用DFS函数进行递归,并输出连通分量。最后,我们在主函数中创建图并调用getConnectedComponents函数。
相关推荐
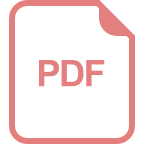
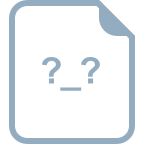
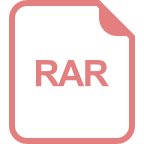
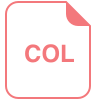
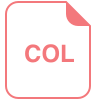
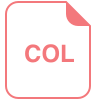
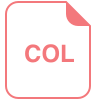
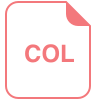









