有什么问题#include<iostream> using namespace std; int main(){ int n,m,count=0; cin>>n; int maxn=-1; int z[108]={0}; for(int i=0;i<n;i++){ cin>>m; z[m]++; } for(int i=0;i<108;i++){ //TODO if(z[i]!=0){ if(z[i]>maxn){ maxn=i; } z[i]--; count++; } } cout<<maxn<<" "<<count; return 0; }
时间: 2024-04-27 14:24:58 浏览: 8
这段代码是一个统计给定数列中出现次数最多的数,并输出该数和数列中所有数出现次数之和的程序。其中,输入的第一个数表示数列中数的个数,接下来的数列中每个数表示一个数的值。程序使用了桶排序的思想,将每个数出现的次数存储在一个长度为108的数组中,最后遍历该数组,找到出现次数最多的数,并计算出数列中所有数的出现次数之和。
需要注意的是,在遍历数组时,若当前元素不为0,则代表该元素对应的数出现过,因此需要将该元素减1,并累加计数器。
相关问题
#include<iostream> #include<algorithm> #include<iomanip> #include<cmath> #include<cstdio> #include<cstring> #include<string> using namespace std; int main() { int count = 0; string a; getline(cin, a); for (int i = 0; i < a.size(); ++i) { /*if (a[i] >= 'A' && a[i] <= 'Z') count++; if (a[i] >= 'a' && a[i] <= 'z') count++; if (a[i] >= '0' && a[i] <= '9') count++;*/ if (a[i] != ' ' && a[i] != '\n') ++count; } cout << count; return 0; }给上面的代码加上注释
```
#include<iostream> // 输入输出流
#include<algorithm> // 算法库
#include<iomanip> // 输入输出格式控制库
#include<cmath> // 数学库
#include<cstdio> // 标准输入输出库
#include<cstring> // 字符串库
#include<string> // 字符串库
using namespace std; // 标准命名空间
int main() { // 主函数开始
int count = 0; // 定义变量count并初始化为0
string a; // 定义字符串变量a
getline(cin, a); // 从标准输入流中读取整行字符串存入变量a中
for (int i = 0; i < a.size(); ++i) // 循环遍历字符串a中的每一个字符
{
/*if (a[i] >= 'A' && a[i] <= 'Z') // 如果字符是大写字母
count++; // 计数器加1
if (a[i] >= 'a' && a[i] <= 'z') // 如果字符是小写字母
count++; // 计数器加1
if (a[i] >= '0' && a[i] <= '9') // 如果字符是数字
count++; // 计数器加1*/
if (a[i] != ' ' && a[i] != '\n') // 如果字符不是空格或换行符
++count; // 计数器加1
}
cout << count; // 输出计数器的值
return 0; // 返回0表示程序正常结束
} // 主函数结束
```
#include <iostream> using namespace std; int main() { int num; while (cin >> num) { int count = 0; while (num) { if (num % 2) { count++; } num /= 2; } cout << count << endl; } return 0; }转为c语言代码
以下是将给定 C++ 代码转换为 C 代码的结果:
```
#include <stdio.h>
int main() {
int num;
while (scanf("%d", &num) != EOF) {
int count = 0;
while (num) {
if (num % 2) {
count++;
}
num /= 2;
}
printf("%d\n", count);
}
return 0;
}
```
请注意,此代码已进行了以下更改:
1. 替换了 iostream 头文件为 stdio.h 头文件。
2. 替换了 cin 输入操作符为 scanf 函数。
3. 替换了 cout 输出操作符为 printf 函数。
4. 移除了 using namespace std。
5. 添加了 EOF 检查以停止循环。
相关推荐
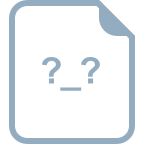
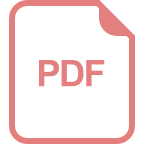
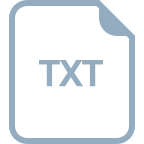













