mapreduce实现对输入文件的排序
时间: 2023-04-30 14:04:03 浏览: 102
MapReduce实现对输入文件的排序,需要以下步骤:
1. Map阶段:将输入文件逐行读入,以每行为单位进行处理。每个Map任务将读入的一行文本按照指定的排序规则进行转换,生成一个键值对,其中键表示排序关键字,值为原始文本行。输出所有生成的键值对。
2. Shuffle阶段:将所有Map任务输出的键值对按照键值进行排序并按照键值分组,将相同键值的键值对存放到同一个Reduce任务中处理。
3. Reduce阶段:将每个Reduce任务中所有键值对按照键进行排序,并将排序后的值部分写入输出文件。
4. 合并输出:将所有Reduce任务输出文件的内容合并生成最终的排序结果文件。
相关问题
请编写MapReduce程序编写程序实现对输入文件的排序
好的,以下是MapReduce程序的实现步骤:
1. Map阶段:将每一行作为一个记录,以该行中的某个字段作为key,整行作为value输出。
2. Shuffle阶段:根据Map输出的key值进行分组,将同一个key值的value分到同一个Reduce Task中。
3. Reduce阶段:对每一个Reduce Task中的记录按照key进行排序,输出排序后的结果。
以下是MapReduce程序的实现代码:
Map阶段:
```java
public static class SortMapper extends Mapper<LongWritable, Text, Text, Text> {
private Text outKey = new Text();
private Text outValue = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] strs = value.toString().split(",");
outKey.set(strs[0]); // 以第一个字段作为key
outValue.set(value.toString());
context.write(outKey, outValue);
}
}
```
Reduce阶段:
```java
public static class SortReducer extends Reducer<Text, Text, Text, NullWritable> {
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
List<String> list = new ArrayList<String>();
for (Text value : values) {
list.add(value.toString());
}
Collections.sort(list); // 对记录按照key进行排序
for (String value : list) {
context.write(new Text(value), NullWritable.get());
}
}
}
```
需要注意的是,以上代码只实现了升序排序,如需实现降序排序,只需将Collections.sort(list)改为Collections.reverse(list)即可。
另外,在提交MapReduce程序之前,需要设置Job的输入路径、输出路径、Mapper和Reducer等信息,以下是完整代码:
```java
public class SortJob {
public static class SortMapper extends Mapper<LongWritable, Text, Text, Text> {
private Text outKey = new Text();
private Text outValue = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] strs = value.toString().split(",");
outKey.set(strs[0]); // 以第一个字段作为key
outValue.set(value.toString());
context.write(outKey, outValue);
}
}
public static class SortReducer extends Reducer<Text, Text, Text, NullWritable> {
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
List<String> list = new ArrayList<String>();
for (Text value : values) {
list.add(value.toString());
}
Collections.sort(list); // 对记录按照key进行排序
for (String value : list) {
context.write(new Text(value), NullWritable.get());
}
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "SortJob");
job.setJarByClass(SortJob.class);
job.setMapperClass(SortMapper.class);
job.setReducerClass(SortReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
mapreduce实现文件合并和去重
### 回答1:
MapReduce实现文件合并和去重可以通过以下步骤完成:
1. Map阶段:读取输入文件并进行分块,每个分块通过一个Map函数处理,生成键-值对,其中键为文件中的某个字段值,值为该字段值对应的行内容。
2. Shuffle阶段:将Map输出的键-值对按照键值进行排序,并按照键值相同的条目聚合为一个列表。
3. Reduce阶段:对每个键值相同的列表进行Reduce操作,将重复的条目删除,并将结果存储到输出文件中。
4. 合并阶段:将所有Reduce输出的文件进行合并,得到最终的文件合并和去重结果。
通过以上步骤,就可以实现MapReduce对文件的合并和去重功能。
### 回答2:
MapReduce是一种用于大数据处理的编程模型和软件框架,它可以将大型数据集分解为若干个小的子集进行并行处理,最终将结果合并。在这样的背景下,MapReduce可以用于实现文件合并和去重的功能。
MapReduce中实现文件合并和去重的步骤如下:
1. 首先,需要将需要被合并的多个小文件划分到多个节点上去处理。
2. 接着,每个节点都会对自己负责的那部分文件进行处理,该处理过程称为Map操作。Map操作将每个小文件中的所有行扫描一遍,将行中的每个单词作为key,当前行所属的文件名作为value输出。
3. 然后,MapReduce框架会将所有的输出进行中间操作,中间操作的目的是既合并相同单词的key,又合并来自不同文件的value。
4. 最后,在Reduce操作中,将中间操作的结果按照key进行汇总,对相同的key进行合并,即将来自不同文件的value合并为一个输出。这其中就包括了去重的操作。
MapReduce在实现文件合并和去重的过程中,利用了分治思想以及并行处理的优势,提高了处理效率。此外,MapReduce中间结果的存储也是基于分布式文件系统的,这样既保证了数据的可靠性,也保证了高并发的处理性能。
### 回答3:
MapReduce是一个用于大数据处理的编程模型,它的基本思想就是将大规模数据分成若干个小块,然后分别对这些小块进行处理,最后将处理结果合并起来。在处理大规模数据时,文件合并和去重是非常常见的需求,接下来我们就来看看MapReduce是如何实现这两个功能的。
首先,我们来看看文件合并。在MapReduce中,文件合并可以分为两个步骤:Map和Reduce。在Map阶段,我们需要将输入文件切分成若干个小块,并将每个小块都映射为一个键值对。键表示该文件块的名称,值表示该文件块的内容。然后,将这些键值对传递给Reduce阶段。在Reduce阶段,我们需要对传入的键值对做一个合并操作,将所有文件块的内容都合并成一个大文件,输出到目标位置。
接下来,我们来看看如何实现去重。在MapReduce中,去重其实就是用一个Set集合来保存已经出现过的字符串,保证不重复。具体的实现步骤如下:在Map阶段,我们将输入文件分成若干个小块,并将每个小块都映射为一个键值对。键表示该文件块的名称,值表示该文件块的内容。然后,对每个文件块进行处理,先将该文件块中的所有字符串拆分出来,然后遍历每个字符串,判断该字符串是否已经出现在Set集合中,如果没有出现过,则将该字符串添加到Set集合中,并将该字符串作为键,一个任意值作为值映射后发送给Reduce节点。在Reduce节点中,我们无需做任何操作,只需将每个键映射的任意值输出即可,这样就实现了去重的功能。
综上所述,MapReduce能够非常方便地实现文件合并和去重的功能,其基于键值对和Map和Reduce两个阶段的编程模型,使得处理大规模数据变得非常简单易用。
相关推荐
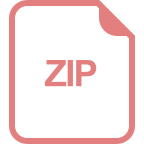
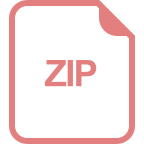












