使用IDEA实现MapReduce时间戳排序
时间: 2024-03-19 07:44:39 浏览: 80
首先,需要在IDEA中创建一个Maven项目,并添加Hadoop的依赖。然后,创建一个Java类来实现MapReduce任务。
下面是实现时间戳排序的MapReduce任务的代码:
```java
import java.io.IOException;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class TimestampSorter {
public static class TimestampMapper extends Mapper<LongWritable, Text, LongWritable, Text> {
private static SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
@Override
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] fields = value.toString().split(",");
String timestampStr = fields[0];
String data = fields[1];
try {
Date timestamp = sdf.parse(timestampStr);
context.write(new LongWritable(timestamp.getTime()), new Text(data));
} catch (ParseException e) {
e.printStackTrace();
}
}
}
public static class TimestampReducer extends Reducer<LongWritable, Text, LongWritable, Text> {
@Override
public void reduce(LongWritable key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
for (Text value : values) {
context.write(key, value);
}
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "timestamp sort");
job.setJarByClass(TimestampSorter.class);
job.setMapperClass(TimestampMapper.class);
job.setReducerClass(TimestampReducer.class);
job.setOutputKeyClass(LongWritable.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
在上面的代码中,我们首先定义了一个Mapper类,将每一行数据的时间戳转换为毫秒级别的long类型,作为Map的输出键,原始数据作为Map的输出值。
然后,定义了一个Reducer类,将Map输出的键值对按照键的大小排序,并将排序后的结果输出到文件中。
最后,在main函数中设置了MapReduce任务的各种参数,包括输入路径、输出路径、Mapper类、Reducer类等,并启动任务。
阅读全文
相关推荐
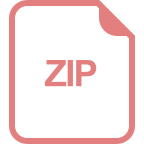
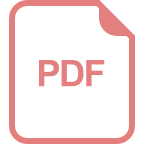














