linux c语言读取程序所在位置的配置文件每一行的内容保存在不同的变量中
时间: 2024-02-01 09:14:24 浏览: 80
可以使用C语言中的标准文件操作函数读取配置文件,然后将读取到的每一行内容保存到不同的变量中。
以下是一个示例代码,假设配置文件名为“config.txt”,包含以下内容:
```
ip=127.0.0.1
port=8080
path=/var/www/html
```
代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 100
int main() {
FILE *fp;
char line[MAX_LINE_LEN];
char *key, *value;
// 打开配置文件
fp = fopen("config.txt", "r");
if (fp == NULL) {
printf("Error: cannot open file.\n");
exit(1);
}
// 逐行读取文件并解析
while (fgets(line, MAX_LINE_LEN, fp) != NULL) {
// 去掉行末的换行符
line[strcspn(line, "\n")] = 0;
// 分离键值对
key = strtok(line, "=");
value = strtok(NULL, "=");
// 根据键名保存值到不同的变量中
if (strcmp(key, "ip") == 0) {
printf("IP address: %s\n", value);
} else if (strcmp(key, "port") == 0) {
printf("Port number: %s\n", value);
} else if (strcmp(key, "path") == 0) {
printf("Path: %s\n", value);
} else {
printf("Error: unknown key %s.\n", key);
}
}
// 关闭文件
fclose(fp);
return 0;
}
```
该代码将配置文件中每一行的键值对解析出来,然后根据键名保存值到不同的变量中。以上述配置文件为例,运行程序后输出如下:
```
IP address: 127.0.0.1
Port number: 8080
Path: /var/www/html
```
阅读全文
相关推荐
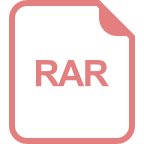









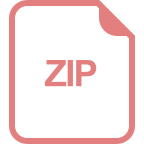
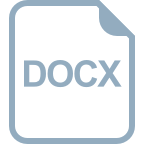
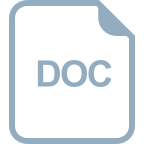
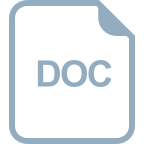
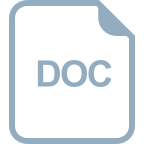
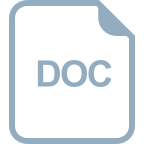
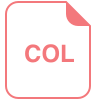