1.题目:分类统计字符个数count_char 2.实验要求:程序接收用户输入的一行字符(字符个数不超过80个,该字符串以回车符结束),并按字母、数字及其它字符分类计数,然后将结果分别显示出来。要求有信息提示用户按照要求输入字符串,三类字符的个数分别在三行显示,并指明是哪类字符。 3.提示:程序可采用input宏或21H号中断的0AH功能调用把输入字符直接送到缓冲区中,然后逐个计数。也可以采用21H号中断的01H功能调用在接收字符后先分类计数然后再存入字符串缓冲区中。
时间: 2024-02-06 12:10:10 浏览: 82
下面是一份 Python 代码,可以实现题目要求的功能:
```python
alpha_count = 0
digit_count = 0
other_count = 0
user_input = input("请输入一行字符(字符个数不超过80个,以回车符结束):")
for char in user_input:
if char.isalpha():
alpha_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
print("字母个数:", alpha_count)
print("数字个数:", digit_count)
print("其它字符个数:", other_count)
```
首先,我们定义了三个变量 `alpha_count`、`digit_count` 和 `other_count`,用于分别统计字母、数字和其它字符的个数。然后,通过 `input` 函数获取用户输入的一行字符,并将其存储在 `user_input` 变量中。
接下来,我们遍历 `user_input` 中的每个字符,使用 `isalpha` 和 `isdigit` 方法来判断该字符是字母还是数字,如果都不是,则认为是其它字符。根据字符的类型,我们分别更新相应的计数器。
最后,我们使用 `print` 函数将三类字符的个数分别输出到屏幕上。
相关问题
python1.统计不同字符个数。用户从键盘输入一行字符,编写一个程序,统计并输出其中英文字符、数字、空格和其他字符的个数。
可以使用Python编写一个程序来实现统计不同字符个数的功能。以下是一个示例代码:
```python
def count_characters(input_string):
# 初始化计数器
count_letters = 0
count_digits = 0
count_spaces = 0
count_others = 0
# 遍历输入字符串的每个字符
for char in input_string:
# 判断字符类型并增加相应计数器的值
if char.isalpha():
count_letters += 1
elif char.isdigit():
count_digits += 1
elif char.isspace():
count_spaces += 1
else:
count_others += 1
# 输出结果
print("英文字符个数:", count_letters)
print("数字个数:", count_digits)
print("空格个数:", count_spaces)
print("其他字符个数:", count_others)
# 从键盘读取输入字符串
input_string = input("请输入一行字符:")
# 调用函数进行统计
count_characters(input_string)
```
运行以上代码,用户可以从键盘输入一行字符,程序将统计并输出其中英文字符、数字、空格和其他字符的个数。
用python编写代码:统计不同字符个数。对于输入的一行字符,统计英文、数字、空格和其他字符的个数
在Python中,你可以使用内置函数以及正则表达式库`re`来统计字符串中不同字符类型的个数。下面是一个简单的示例:
```python
import re
def count_chars(input_string):
# 定义一个字典来存储每种字符类型的计数
char_count = {
'English': 0,
'Numbers': 0,
'Spaces': 0,
'Others': 0
}
# 使用正则表达式匹配英文字符、数字、空格和其他字符
for match in re.findall(r"[\w\s]", input_string):
if match.isalpha():
char_count['English'] += 1
elif match.isdigit():
char_count['Numbers'] += 1
elif match.isspace():
char_count['Spaces'] += 1
else:
char_count['Others'] += 1
return char_count
# 测试这个函数
input_string = "Hello World! 123 Test."
print(count_chars(input_string))
阅读全文
相关推荐
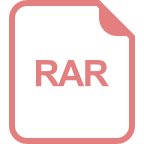
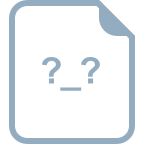
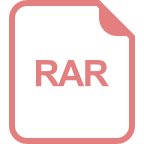













