AESRSA混合加密系统代码
时间: 2023-10-15 14:02:38 浏览: 45
以下是一个基于Python的AES-RSA混合加密系统的示例代码:
```python
import os
import base64
from Crypto.Cipher import AES
from Crypto.PublicKey import RSA
from Crypto.Random import get_random_bytes
# 生成随机密钥
def generate_key():
return get_random_bytes(16)
# AES加密
def aes_encrypt(message, key):
iv = get_random_bytes(AES.block_size)
cipher = AES.new(key, AES.MODE_CBC, iv)
ciphertext = cipher.encrypt(message)
return iv + ciphertext
# AES解密
def aes_decrypt(ciphertext, key):
iv = ciphertext[:AES.block_size]
cipher = AES.new(key, AES.MODE_CBC, iv)
plaintext = cipher.decrypt(ciphertext[AES.block_size:])
return plaintext
# RSA加密
def rsa_encrypt(message, public_key):
rsa_key = RSA.import_key(public_key)
ciphertext = rsa_key.encrypt(message, None)[0]
return base64.b64encode(ciphertext)
# RSA解密
def rsa_decrypt(ciphertext, private_key):
rsa_key = RSA.import_key(private_key)
ciphertext = base64.b64decode(ciphertext)
plaintext = rsa_key.decrypt(ciphertext)
return plaintext
# 加密函数
def encrypt(message, public_key):
# 生成AES密钥
key = generate_key()
# AES加密
ciphertext = aes_encrypt(message.encode(), key)
# RSA加密AES密钥
encrypted_key = rsa_encrypt(key, public_key)
return ciphertext, encrypted_key
# 解密函数
def decrypt(ciphertext, encrypted_key, private_key):
# RSA解密AES密钥
key = rsa_decrypt(encrypted_key, private_key)
# AES解密
plaintext = aes_decrypt(ciphertext, key)
return plaintext.decode()
# 测试
message = 'Hello, world!'
key = RSA.generate(2048)
public_key = key.publickey().export_key()
private_key = key.export_key()
ciphertext, encrypted_key = encrypt(message, public_key)
plaintext = decrypt(ciphertext, encrypted_key, private_key)
print('原文:', message)
print('密文:', ciphertext)
print('密钥:', encrypted_key)
print('解密后:', plaintext)
```
在上述代码中,首先定义了四个函数,分别用于生成随机密钥、AES加密、AES解密和RSA加密、RSA解密。其中,AES使用CBC模式,RSA使用PKCS#1 OAEP填充方式。
然后,定义了一个`encrypt`函数和一个`decrypt`函数,用于分别加密和解密数据。其中,`encrypt`函数首先生成一个随机的AES密钥,然后使用该密钥对数据进行AES加密,最后使用RSA公钥加密AES密钥。`decrypt`函数则是对加密过程的逆向操作,先使用RSA私钥解密AES密钥,再用解密得到的AES密钥对数据进行AES解密。
最后,在测试部分,使用RSA生成一个公私钥对,将明文加密并输出密文、加密后的AES密钥、解密后的明文。
相关推荐
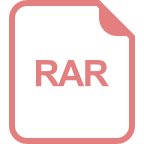














