C++编程实现浮点数转ieee754
时间: 2023-06-09 17:05:00 浏览: 98
可以使用联合体(union)来实现浮点数转换为IEEE 754标准格式的字节序列。
下面是一个示例代码:
```c
#include <stdio.h>
#include <stdint.h>
union FloatConverter {
float f;
uint32_t u;
};
void float2bytes(float f, uint8_t bytes[4]) {
union FloatConverter converter;
converter.f = f;
bytes[0] = (converter.u >> 24) & 0xFF;
bytes[1] = (converter.u >> 16) & 0xFF;
bytes[2] = (converter.u >> 8) & 0xFF;
bytes[3] = converter.u & 0xFF;
}
int main() {
float f = -123.456f;
uint8_t bytes[4];
float2bytes(f, bytes);
printf("Float: %f\n", f);
printf("Bytes: 0x%02X 0x%02X 0x%02X 0x%02X\n", bytes[0], bytes[1], bytes[2], bytes[3]);
return 0;
}
```
此代码将浮点数 `-123.456f` 转换为 4 个字节的字节序列,并输出结果:
```
Float: -123.456001
Bytes: 0xC2 0xF5 0x28 0xC6
```
注意,由于浮点数是有限精度的,所以在转换后再将字节序列转换回原始浮点数时可能会存在精度损失。
阅读全文
相关推荐
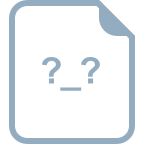

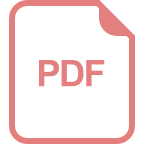
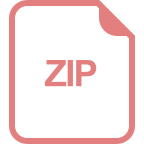
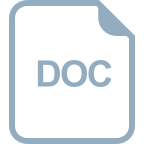
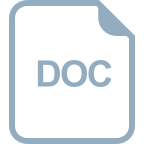
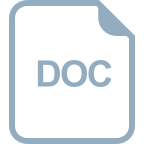
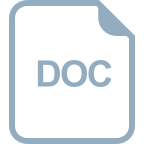
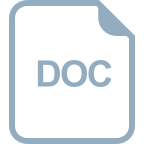
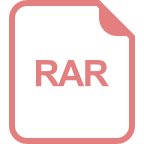
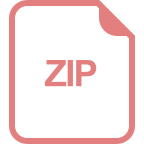
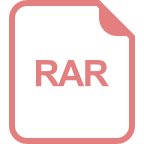
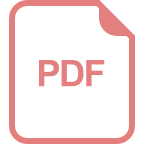
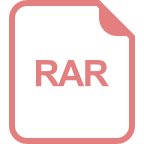
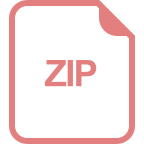
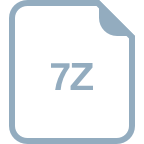