解释public NewInfor selectById(Integer id) { NewInfor newsInfor = new NewInfor(); //��ѯ���е����� String sql = "select * from news_infor where id=?"; try { // ��ѯ ps = conn.prepareStatement(sql); ps.setInt(1, id); rs = ps.executeQuery(); // ��װ���� while (rs.next()) { newsInfor.setId(rs.getInt(1)); newsInfor.setTitle(rs.getString(2)); newsInfor.setContent(rs.getString(3)); newsInfor.setAuthor(rs.getString(4)); newsInfor.setType(rs.getString(5)); newsInfor.setTime(rs.getTimestamp(6)); } }
时间: 2024-02-14 08:35:19 浏览: 116
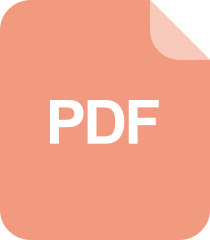
关于使用Mybatisplus自带的selectById和insert方法时的一些问题
这是一个在数据库中查询指定 id 的新闻信息的方法。方法的实现步骤如下:
1. 创建一个 NewInfor 对象作为返回值。
2. 构造 SQL 语句,使用占位符 "?" 表示需要传入参数的位置。
3. 创建 PreparedStatement 对象,并将 SQL 语句中的占位符替换为具体的参数值。
4. 执行 SQL 查询,返回 ResultSet 对象。
5. 遍历 ResultSet 对象,将查询结果赋值给 NewInfor 对象的属性。
6. 返回 NewInfor 对象。
注释中给出了每个步骤的解释。这个方法的作用是返回具有指定 id 的新闻信息,方法的返回值是一个 NewInfor 对象,包含了从数据库中查询到的新闻信息。
阅读全文
相关推荐
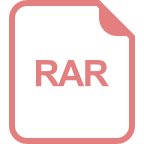
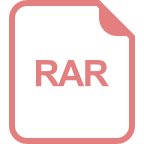
















