用Java求解编写一个程序要求返回在BST中第k小的元素全过程。
时间: 2024-03-09 08:46:43 浏览: 34
好的,下面是求解BST中第k小元素的Java程序:
```java
class Node {
int val;
Node left, right;
public Node(int val) {
this.val = val;
left = null;
right = null;
}
}
class BST {
Node root;
public BST() {
root = null;
}
// 插入节点
public void insert(int val) {
root = insertNode(root, val);
}
// 递归插入节点
public Node insertNode(Node root, int val) {
if (root == null) {
return new Node(val);
}
if (val < root.val) {
root.left = insertNode(root.left, val);
} else {
root.right = insertNode(root.right, val);
}
return root;
}
// 返回第k小的元素
public int kthSmallest(int k) {
int[] res = new int[2];
inorder(root, k, res);
return res[1];
}
// 中序遍历
public void inorder(Node root, int k, int[] res) {
if (root == null) {
return;
}
inorder(root.left, k, res);
if (++res[0] == k) {
res[1] = root.val;
return;
}
inorder(root.right, k, res);
}
}
public class Main {
public static void main(String[] args) {
BST bst = new BST();
bst.insert(5);
bst.insert(3);
bst.insert(6);
bst.insert(2);
bst.insert(4);
bst.insert(1);
int k = 3;
System.out.println("第" + k + "小的元素为:" + bst.kthSmallest(k));
}
}
```
在这个程序中,我们先定义了一个`Node`类表示二叉搜索树的节点,其中包括节点值和左右子树。然后我们定义了一个`BST`类表示二叉搜索树,其中包括根节点和插入节点方法。插入节点方法采用递归实现,将新节点插入二叉搜索树中。最后,我们实现了一个`kthSmallest`方法,用于返回二叉搜索树中第k小的元素。该方法采用中序遍历实现,当遍历到第k个节点时,返回该节点的值即可。
在`main`函数中,我们先创建了一个二叉搜索树,并插入了一些节点。然后设置k为3,调用`kthSmallest`方法并输出结果。
希望这个程序能够帮到你,如果你还有其他问题请随时问我!
相关推荐
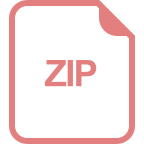














