python利用八叉树分割三维点云代码
时间: 2023-10-21 09:19:23 浏览: 189
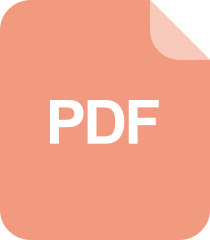
基于三维点云数据的线性八叉树编码压缩算法

以下是利用八叉树分割三维点云的Python代码示例:
```python
import numpy as np
from sklearn.neighbors import KDTree
class Octree:
def __init__(self, points, max_points_per_node=16, max_depth=8):
self.points = points
self.max_points_per_node = max_points_per_node
self.max_depth = max_depth
self.min_bounds = np.min(points, axis=0)
self.max_bounds = np.max(points, axis=0)
self.center = (self.min_bounds + self.max_bounds) / 2
self.children = None
self.depth = 0
if len(points) > max_points_per_node and max_depth > 0:
self.split()
def split(self):
self.children = []
for i in range(8):
mask = np.array([i & 1, i & 2, i & 4])
bounds_min = np.where(mask, self.center, self.min_bounds)
bounds_max = np.where(mask, self.max_bounds, self.center)
indices = self.get_indices(bounds_min, bounds_max)
if len(indices) > 0:
child = Octree(self.points[indices], self.max_points_per_node, self.max_depth - 1)
child.min_bounds = bounds_min
child.max_bounds = bounds_max
child.depth = self.depth + 1
self.children.append(child)
def get_indices(self, min_bounds, max_bounds):
indices = []
for i, point in enumerate(self.points):
if (point >= min_bounds).all() and (point <= max_bounds).all():
indices.append(i)
return indices
def get_points_in_sphere(self, center, radius):
indices = self.get_indices(center - radius, center + radius)
if self.children is None:
return indices
else:
result = []
for child in self.children:
if (center - child.center).dot(center - child.center) < radius * radius:
result += child.get_points_in_sphere(center, radius)
return result
def get_points_in_box(self, min_bounds, max_bounds):
indices = self.get_indices(min_bounds, max_bounds)
if self.children is None:
return indices
else:
result = []
for child in self.children:
if (min_bounds <= child.max_bounds).all() and (max_bounds >= child.min_bounds).all():
result += child.get_points_in_box(min_bounds, max_bounds)
return result
def get_points_in_cube(self, center, size):
return self.get_points_in_box(center - size / 2, center + size / 2)
def octree_from_points(points, max_points_per_node=16, max_depth=8):
return Octree(points, max_points_per_node, max_depth)
def octree_from_kd_tree(kd_tree, max_points_per_node=16, max_depth=8):
return Octree(kd_tree.data, max_points_per_node, max_depth)
def octree_from_file(filename, max_points_per_node=16, max_depth=8):
points = np.loadtxt(filename)
return Octree(points, max_points_per_node, max_depth)
def kd_tree_from_points(points):
return KDTree(points)
def kd_tree_from_file(filename):
points = np.loadtxt(filename)
return KDTree(points)
```
这个Octree类实现了八叉树的基本功能,包括分割点云、获取半径内的点、获取包含在指定盒子中的点等。可以使用octree_from_points、octree_from_kd_tree和octree_from_file函数来创建Octree对象。也可以使用kd_tree_from_points和kd_tree_from_file函数来创建KDTree对象。
阅读全文
相关推荐
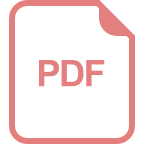
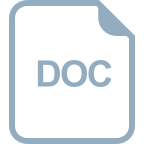
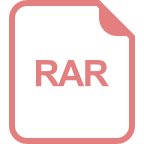
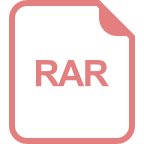
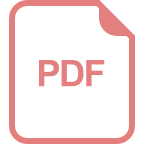
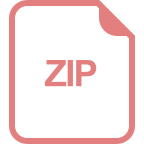
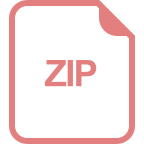
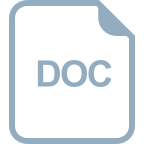
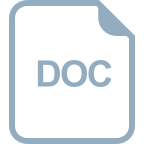
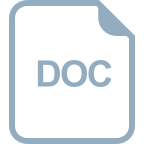
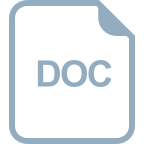
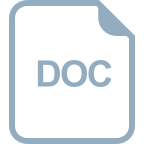
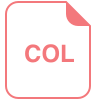
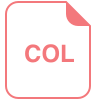
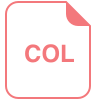
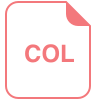
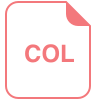